GoLang Tutorial - Arrays and Slices
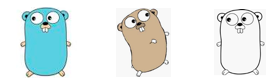
The slice type is an abstraction built on top of Go's array type, and so to understand slices we must first understand arrays.
An array variable denotes the entire array; unlike C, array name is not a pointer to the first array element. This means that when we assign or pass around an array value we will make a copy of its contents. (To avoid the copy you could pass a pointer to the array, but then that's a pointer to an array, not an array.
Here is an example of an array:
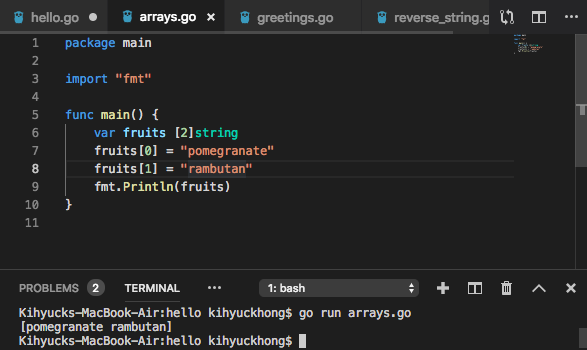
Note that we can declare and assign at the same time:
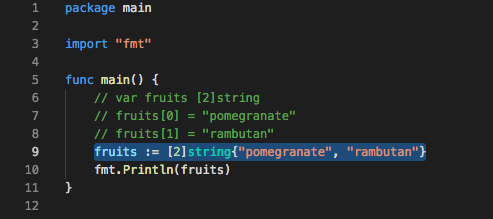
Or like this:
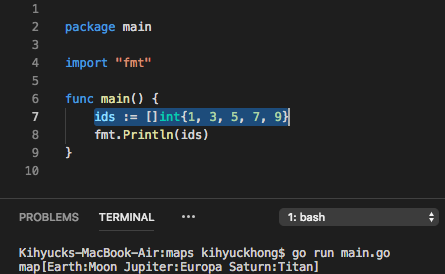
Slices are where the action is, but to use them well one must understand exactly what they are and what they do.
We don't see arrays too often in Go code. Slices, though, are everywhere. They build on arrays to provide great power and convenience.
The type specification for a slice is []T, where T is the type of the elements of the slice. Unlike an array type, a slice type has no specified length.
A slice is a data structure describing a contiguous section of an array stored separately from the slice variable itself. A slice is not an array but it describes a piece of an array.
A slice literal is declared just like an array literal, except we leave out the element count:
alphabet := []string{"a", "b", "c", "d"}
A slice has both a length and a capacity. The length of a slice is the number of elements it contains. The capacity of a slice is the number of elements in the underlying array, counting from the first element in the slice. The length and capacity of a slice s can be obtained using the expressions len(s) and cap(s):
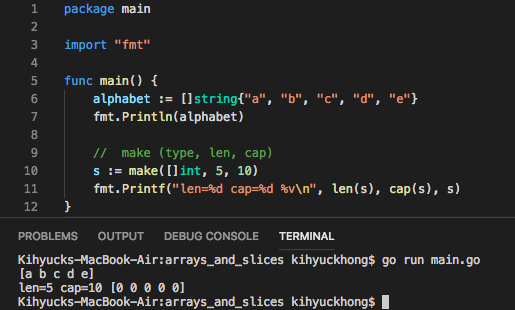
When the capacity argument is omitted, it defaults to the specified length. Here's a more succinct version of the same code:
s := make([]int, 5)
The length and capacity of a slice can be inspected using the built-in len and cap functions as shown in the previous example.
len(s) == 5 cap(s) == 5
Another sample code:
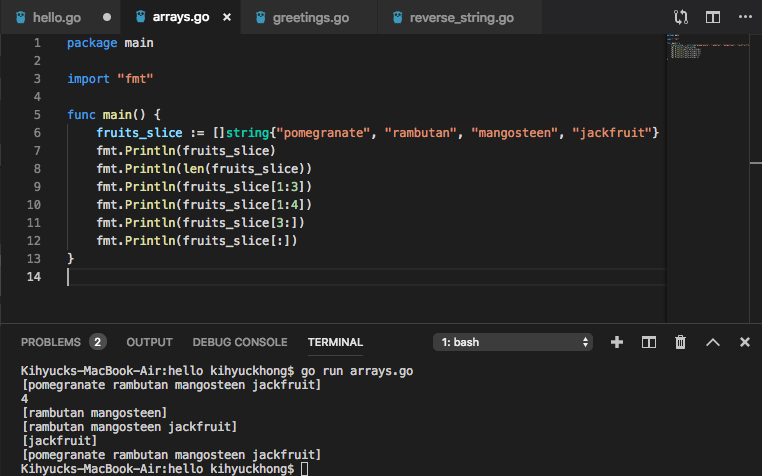
In this section, we'll create a byte slice from a string literal "abc" and append a byte to the byte slice. Then, we convert the byte slice into a string with the string() built-in method. A byte slice has a length, which we retrieve with len. Also, we can access individual bytes. Here is the code:
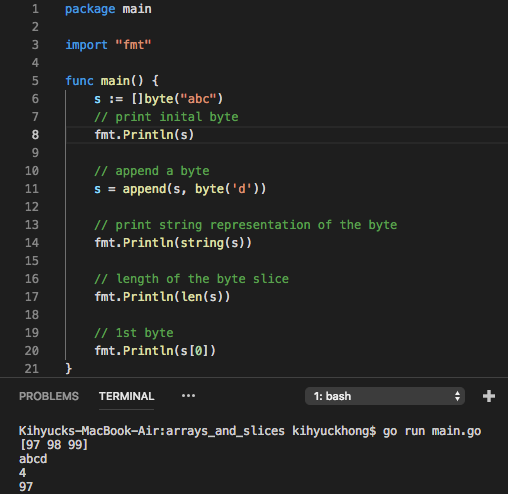
Want to play with arrays and slices? Check this : Arrays vs Slices with an array left rotation sample.
For more on slice - Go Slices: usage and internals
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization