$http - XMLHttpRequest object & json
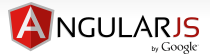
$http in AngularJS is a core service for reading data from web servers.
$http.get(url) is the function to use for reading server data.
<head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-app="myApp" ng-controller="planetController"> <ul> [name], [earth-sun=1], [light speed] <li ng-repeat="x in names"> {{ x.name + ', ' + x.distance + ', ' + x.light }} </li> </ul> </div> <script> var app = angular.module('myApp', []); app.controller('planetController', function($scope, $http) { $http.get("http://www.bogotobogo.com/AngularJS/files/httpRequest/planet2.json") .success(function(response) {$scope.names = response;}); }); </script> </body> </html>
With planet2.json:
[ {"name":"Neptune", "distance":30.087, "light":"4 hours"}, {"name":"Uranus", "distance":19.208, "light":"2 hours 40 minutes"}, {"name":"Saturn", "distance":9.523, "light":"1 hour 20 minutes"}, {"name":"Jupiter", "distance":5.203, "light":"43 minutes"}, {"name":"Mars", "distance":1.524, "light":"12.6 minutes"}, {"name":"Earth", "distance":1.0, "light":"0"}, {"name":"Venus", "distance":0.723, "light":"6 minutes"}, {"name":"Mercury", "distance":0.387, "light":"3 minutes"}, {"name":"Moon", "distance":0.003, "light":"1.5sec"} ]
The AngularJS application is defined by ng-app. The application runs inside a <div>. The ng-controller directive names the controller object. The planetController function is a standard JavaScript object constructor.
AngularJS will invoke planetController with a $scope and $http object. $scope is the application object (the owner of application variables and functions). $http is an XMLHttpRequest object for requesting external data. Then,
$http.get() reads static JSON data from
http://www.bogotobogo.com/AngularJS/files/httpRequest/planet.json.
Note that unlike the case when we use *.js, we need quotes for the keys, so:
{"name":"Neptune", "distance":30.087, "light":"4 hours"},
but not this:
{name:"Neptune", distance:30.087, light:"4 hours"},
If successful, the controller creates a property (names) in the scope, with JSON data from the server.
Recently, scientists found hundreds of galaxies that have been hidden behind our hazy Milkyway galaxy.
These days including the last two decades, without any doubt, astrophysicists are enjoying renaissance. Hubble space telescope's deep field contributions since 1993, and the new one called James Webb infrared space telescope which will be stationed at 4x of the moon's distance in 2018. On top of that, they have another weapon, gravity wave!
They are now talking about "precision": what a big change. Also, we may be on the verge of Grand Unified Theory thanks to the m-theory which may explain big bang as well as singularity physics of blackhole.
I wish I could join them rather than fighting with miniscule bugs!
Anyway, this sample is almost the same as the one in the previous section, but I played a simulation pretending we're communicating with the newly found galaxies:
we get the message from them, and then they also send images to us.
So, in terms of using Angular's $http, not much different from the other sample.
Here is the code:
<!DOCTYPE html> <html> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <body> <div ng-app="myApp" ng-controller="galaxyCtrlA"> <p>The $http service requests responses from the the server on the "Zone of Avoidance" galaxies, and the response are set as the values of the "message" and "pictures" variables.</p> <p>Welcome message from the "Zone of Avoidance" galaxies is:</p> <h1>{{ message }}</h1> <p>The pictures from the "Zone of Avoidance" are:</p> <ul> <br> <li ng-repeat="p in pictures"> <img src="{{ p.image }}" alt="{{ p.image }}" width="60%"> </li> </ul> </div> <script> var app = angular.module('myApp', []); app.controller('galaxyCtrlA', function($scope, $http) { $http.get("http://www.bogotobogo.com/AngularJS/files/httpRequest/welcome.html") .then(function(response) { $scope.message = response.data; }); $http.get("http://www.bogotobogo.com/AngularJS/files/httpRequest/picture-list.json") .success(function(response) { $scope.pictures = response; }); }); </script> </body> </html>;
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization