Bottle micro web services framework 12 : forms get/post II
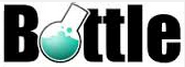
List of Bottle Micro Web Services Tutorials
- Introduction
- Static files
- Template
- json
- Bucket List App I - sqlite, route, and template
- Bucket List App II - get & post
- Bucket List App III - Editing
- Bucket List App IV - route validation, regex, and static_file
- Bucket List App V - json
- json to html table
- Forms - Get & Post
- Forms - Get & Post with editable and checkbox table cells
Continued from Forms - Get & Post.
Here is a slightly samples from the previous chapter:
disp.py:
from bottle import route, run, template, request try: import simplejson as json except ImportError: import json HOST = '192.168.47.101' test_list = [ { 'protocol': 'proto1', 'service':'s1', 'plugin': 'plug1', 'value':1 }, { 'protocol': 'proto2', 'service':'s2', 'plugin': 'plug2', 'value':2 }, { 'protocol': 'proto3', 'service':'s3', 'plugin': 'plug3', 'value':3 }, ] number_of_test_cases = len(test_list) @route('/page1') def serve_homepage(): return template('disp_table',rows = test_list, cases = number_of_test_cases) @route('/new') def add_new(): return template('add_case_post') @route('/new', method='POST') def add_new(): p = request.forms.get('protocol') with open('test.json', 'w') as f: json.dump(test_list, f) print 'p=',p run(host=HOST, port=8080, debug=True)
disp_table.tpl:
%# disp_table.tpl <p>The items are as follows:</p> <table border="1"> <tr> %for subr in rows[0]: <th>{{subr}}</th> %end </tr> %for r in rows: <tr> %for subr in r: <td>{{r[subr]}}</td> %end </tr> %end </table>
add_case.tpl:
<p>Add a new case to the list:</p> <form action="/new" method="GET"> protocol: <input type="text" size="50" maxlength="50" name="protocol"><br> service: <input type="text" size="50" maxlength="50" name="service"><br> plugin: <input type="text" size="50" maxlength="100" name="plugin"><br> value: <input type="text" size="50" maxlength="100" name="value"><br> <input type="submit" name="add" value="Add to the list"> </form>
add_case_post.tpl:
Add a new case to the list:
<p>Add a new case to the list:</p> <form action="/new" method="POST"> protocol: <input type="text" size="50" maxlength="50" name="protocol"><br> service: <input type="text" size="50" maxlength="50" name="service"><br> plugin: <input type="text" size="50" maxlength="100" name="plugin"><br> value: <input type="text" size="50" maxlength="100" name="value"><br> <input type="submit" name="add" value="Add to the list"> </form>
If we run the bottle server:
$ python disp.py Bottle v0.12.7 server starting up (using WSGIRefServer())... Listening on http://192.168.47.101:8080/ Hit Ctrl-C to quit.
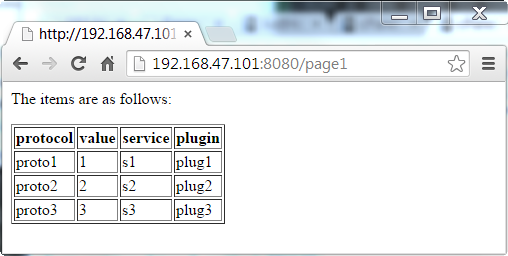
The following code is almost the same as in the previous section except it passes the key for the editable cell:
@route('/page1') def serve_homepage(): return template('disp_table', rows = test_list, cases = number_of_test_cases, edit = 'value')
Note the part: edit = 'value':
This is the full code:
disp.py:
from bottle import route, run, template, request try: import simplejson as json except ImportError: import json HOST = '192.168.47.101' test_list = [ { 'protocol': 'proto1', 'service':'s1', 'plugin': 'plug1', 'value':1 }, { 'protocol': 'proto2', 'service':'s2', 'plugin': 'plug2', 'value':2 }, { 'protocol': 'proto3', 'service':'s3', 'plugin': 'plug3', 'value':3 }, ] number_of_test_cases = len(test_list) @route('/page1') def serve_homepage(): return template('disp_table',rows = test_list, cases = number_of_test_cases) @route('/new') def add_new(): return template('add_case_post') @route('/new', method='POST') def add_new(): p = request.forms.get('protocol') with open('test.json', 'w') as f: json.dump(test_list, f) print 'p=',p run(host=HOST, port=8080, debug=True)
The following table makes a cell editable by setting an attribute 'contenteditable="true" of 'td' element if the key is passed as 'edit':
disp_table.tpl:
%# disp_table.tpl <p>The items are as follows:</p> <table border="1"> <tr> % for subr in rows[0]: <th>{{subr}}</th> % end </tr> % for r in rows: <tr> % for subr in r: % if subr == edit: <td contenteditable="true">{{r[subr]}}</td> % else: <td>{{r[subr]}}</td> % end % end </tr> % end </table>
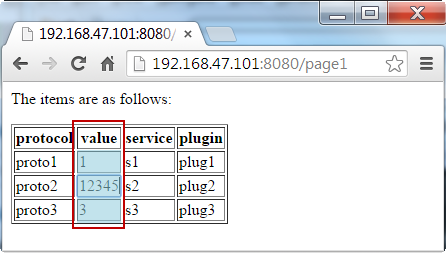
The following code is almost the same as in the previous section except it passes the key for a cell with checkbox:
@route('/page1') def serve_homepage(): return template('disp_table', rows = test_list, cases = number_of_test_cases, edit = 'value', check = 'run')
Note the part: check = 'run' and as we can see from the code below, we added an additional field for checkbox, 'run':
This is the full code:
disp.py:
from bottle import route, run, template, request try: import simplejson as json except ImportError: import json HOST = '192.168.47.101' test_list = [ { 'protocol': 'proto1', 'service':'s1', 'plugin': 'plug1', 'value':1, 'run':1 }, { 'protocol': 'proto2', 'service':'s2', 'plugin': 'plug2', 'value':2, 'run':0 }, { 'protocol': 'proto3', 'service':'s3', 'plugin': 'plug3', 'value':3, 'run':1 }, ] number_of_test_cases = len(test_list) @route('/page1') def serve_homepage(): return template('disp_table', rows = test_list, cases = number_of_test_cases, edit = 'value', check = 'run') @route('/new') def add_new(): return template('add_case_post') @route('/new', method='POST') def add_new(): p = request.forms.get('protocol') with open('test.json', 'w') as f: json.dump(test_list, f) print 'p=',p run(host=HOST, port=8080, debug=True)
The following table makes a cell editable by setting an attribute 'contenteditable="true" of 'td' element if the key is passed as 'edit':
disp_table.tpl:
%# disp_table.tpl <p>The items are as follows:</p> <table border="1"> <tr> % for subr in rows[0]: <th>{{subr}}</th> % end </tr> % for r in rows: <tr> % for subr in r: % if subr == edit: <td contenteditable="true">{{r[subr]}}</td> % elif subr == check: <td> % if r[subr] == 1: <input type="checkbox" name="myTextEditBox" value="1" checked="checked" /> % else: <input type="checkbox" name="myTextEditBox" value="0" /> % end </td> % else: <td>{{r[subr]}}</td> % end % end </tr> % end </table>
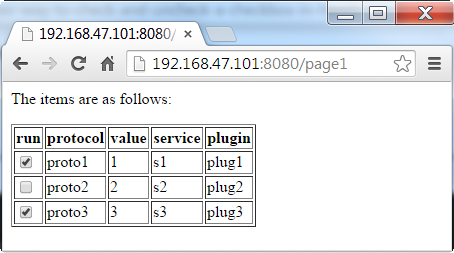
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization