Terraform Tutorial - AWS IAM user, group, role, and policies - part 2
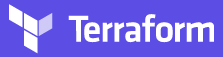
Continued from AWS IAM user, group, role, and policies - part 1, in this post, we'll will create a group and add an IAM user to the group.
main.tf:
terraform { required_providers { aws = { source = "hashicorp/aws" version = "3.42.0" } } } provider "aws" { region = var.region } resource "aws_iam_user" "bogo_user" { name = "bogo_user_1" } resource "aws_iam_access_key" "my_access_key" { user = aws_iam_user.bogo_user.name pgp_key = var.pgp_key } resource "random_pet" "pet_name" { length = 3 separator = "-" } resource "aws_s3_bucket" "bogo_bucket" { bucket = "${random_pet.pet_name.id}-bucket" acl = "private" tags = { Name = "My bucket" Environment = "Dev" } } data "aws_iam_policy_document" "s3_policy" { statement { actions = ["s3:ListAllMyBuckets"] resources = ["arn:aws:s3:::*"] effect = "Allow" } statement { actions = ["s3:*"] resources = [aws_s3_bucket.bogo_bucket.arn] effect = "Allow" } } resource "aws_iam_policy" "bogo_policy" { name = "${random_pet.pet_name.id}-policy" description = "My test policy" policy = data.aws_iam_policy_document.s3_policy.json } resource "aws_iam_group" "bogo_admin_group" { name = "BogoAdmin" } resource "aws_iam_group_policy_attachment" "custom_policy" { group = aws_iam_group.bogo_admin_group.name policy_arn = aws_iam_policy.bogo_policy.arn } resource "aws_iam_group_membership" "team" { name = "tf-testing-group-membership" users = [ aws_iam_user.bogo_user.name, ] group = aws_iam_group.bogo_admin_group.name } output "rendered_policy" { value = data.aws_iam_policy_document.s3_policy.json } output "secret" { value = aws_iam_access_key.my_access_key.encrypted_secret sensitive = true }
variables.tf:
variable "region" { default = "us-east-1" } variable "pgp_key" { description = "Either a base-64 encoded PGP public key, or a keybase username in the form keybase:username. Used to encrypt the password and the access key on output to the console." default = "" }
Before anything else, we need to do terraform init
to Initializing the backend and provider plugins.
$ terraform init Initializing the backend... Initializing provider plugins... - Reusing previous version of hashicorp/aws from the dependency lock file - Using previously-installed hashicorp/aws v3.42.0 Terraform has been successfully initialized ...
Now, run terraform plan
and terraform apply
commands:
$ terraform plan $ terraform apply --auto-approve random_pet.pet_name: Creating... random_pet.pet_name: Creation complete after 0s [id=physically-immortal-pika] aws_iam_group.bogo_admin_group: Creating... aws_iam_user.bogo_user: Creating... aws_s3_bucket.bogo_bucket: Creating... aws_iam_group.bogo_admin_group: Creation complete after 1s [id=BogoAdmin] aws_iam_user.bogo_user: Creation complete after 1s [id=bogo_user_1] aws_iam_access_key.my_access_key: Creating... aws_iam_group_membership.team: Creating... aws_iam_access_key.my_access_key: Creation complete after 1s [id=AKIAQV57YS3YMNUW7N3H] aws_iam_group_membership.team: Creation complete after 1s [id=tf-testing-group-membership] aws_s3_bucket.bogo_bucket: Creation complete after 7s [id=physically-immortal-pika-bucket] data.aws_iam_policy_document.s3_policy: Reading... data.aws_iam_policy_document.s3_policy: Read complete after 0s [id=583517222] aws_iam_policy.bogo_policy: Creating... aws_iam_policy.bogo_policy: Creation complete after 2s [id=arn:aws:iam::047109936880:policy/physically-immortal-pika-policy] aws_iam_group_policy_attachment.custom_policy: Creating... aws_iam_group_policy_attachment.custom_policy: Creation complete after 1s [id=BogoAdmin-20210528165109510000000001] Apply complete! Resources: 8 added, 0 changed, 0 destroyed. Outputs: rendered_policy = <<EOT { "Version": "2012-10-17", "Statement": [ { "Sid": "", "Effect": "Allow", "Action": "s3:ListAllMyBuckets", "Resource": "arn:aws:s3:::*" }, { "Sid": "", "Effect": "Allow", "Action": "s3:*", "Resource": "arn:aws:s3:::physically-immortal-pika-bucket" } ] } EOT secret = <sensitive>
We can see the group and a user in that group have been created:
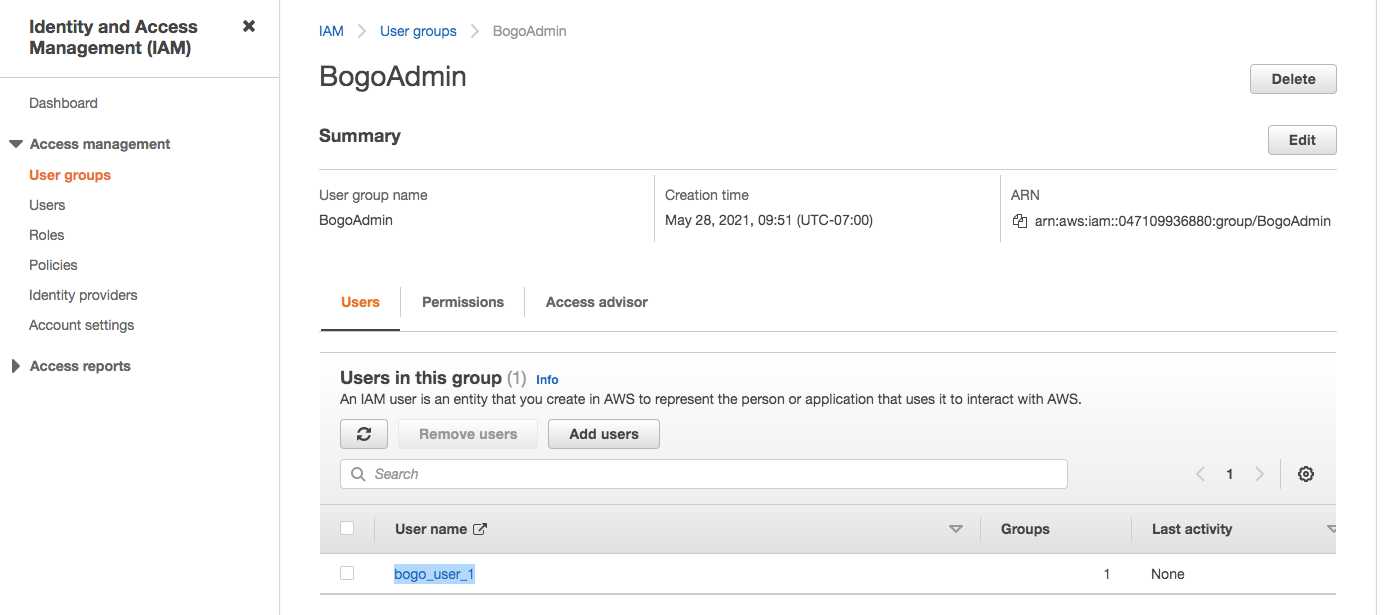
Similar to the previous sections, but this time we'll add 3 users to the group.
main.tf:
terraform { required_providers { aws = { source = "hashicorp/aws" version = "3.42.0" } } } provider "aws" { region = var.region } resource "aws_iam_user" "bogo_users" { for_each = toset(var.user_names) # note: each.key and each.value are the same for a set name = each.value } resource "aws_iam_access_key" "my_access_key" { for_each = aws_iam_user.bogo_users user = each.value.name pgp_key = var.pgp_key } resource "random_pet" "pet_name" { length = 3 separator = "-" } resource "aws_s3_bucket" "bogo_bucket" { bucket = "${random_pet.pet_name.id}-bucket" acl = "private" tags = { Name = "My bucket" Environment = "Dev" } } data "aws_iam_policy_document" "s3_policy" { statement { actions = ["s3:ListAllMyBuckets"] resources = ["arn:aws:s3:::*"] effect = "Allow" } statement { actions = ["s3:*"] resources = [aws_s3_bucket.bogo_bucket.arn] effect = "Allow" } } resource "aws_iam_policy" "bogo_policy" { name = "${random_pet.pet_name.id}-policy" description = "My test policy" policy = data.aws_iam_policy_document.s3_policy.json } resource "aws_iam_group" "bogo_admin_group" { name = "BogoAdmin" } resource "aws_iam_group_policy_attachment" "custom_policy" { group = aws_iam_group.bogo_admin_group.name policy_arn = aws_iam_policy.bogo_policy.arn } resource "aws_iam_group_membership" "team" { name = "tf-testing-group-membership" users = var.user_names group = aws_iam_group.bogo_admin_group.name } output "rendered_policy" { value = data.aws_iam_policy_document.s3_policy.json }
variables.tf:
variable "region" { default = "us-east-1" } variable "pgp_key" { description = "Either a base-64 encoded PGP public key, or a keybase username in the form keybase:username. Used to encrypt the password and the access key on output to the console." default = "keybase:bogotobogo" } variable "user_names" { description = "Create IAM users with these names" type = list(string) default = ["bogo_user_1", "bogo_user_2", "bogo_user_3"] }
After terraform apply
, we can see the outcome.
Three users are under BogoAdmin group:
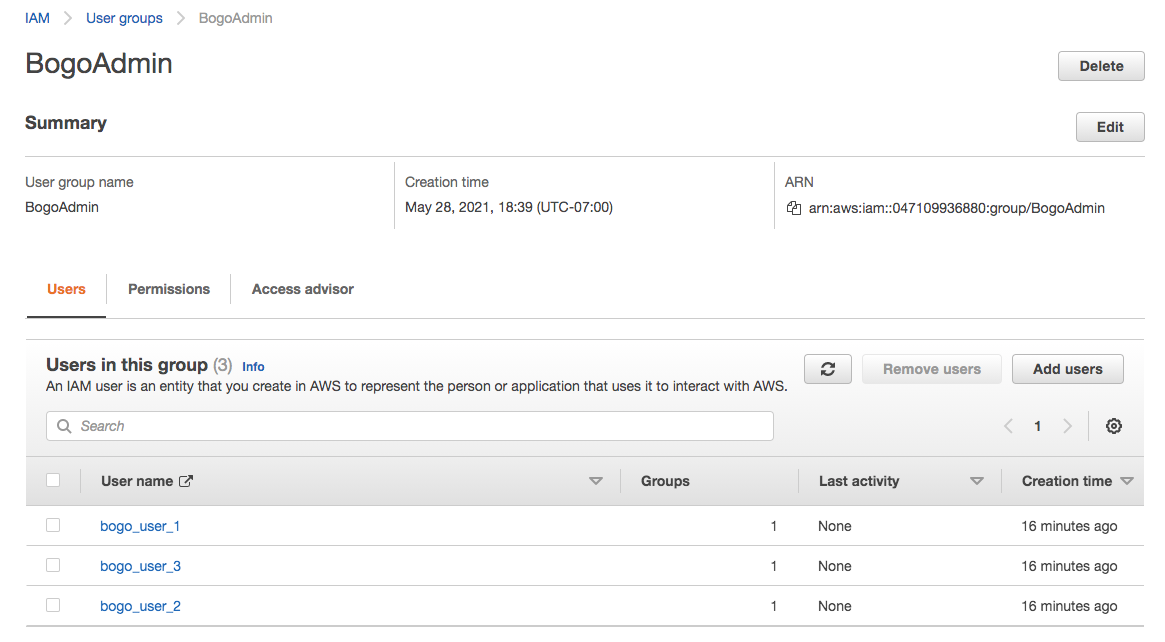
We can check the credentials (access key & secret) are created for user_3:
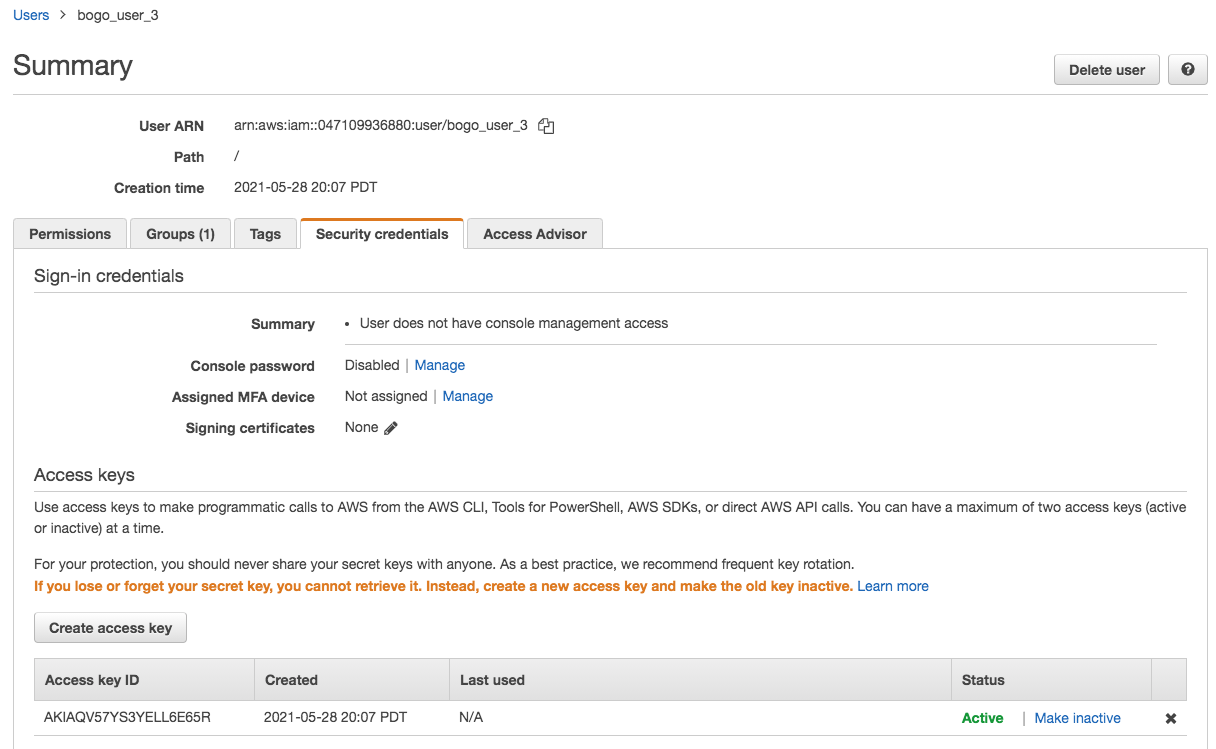
S3 bucket:
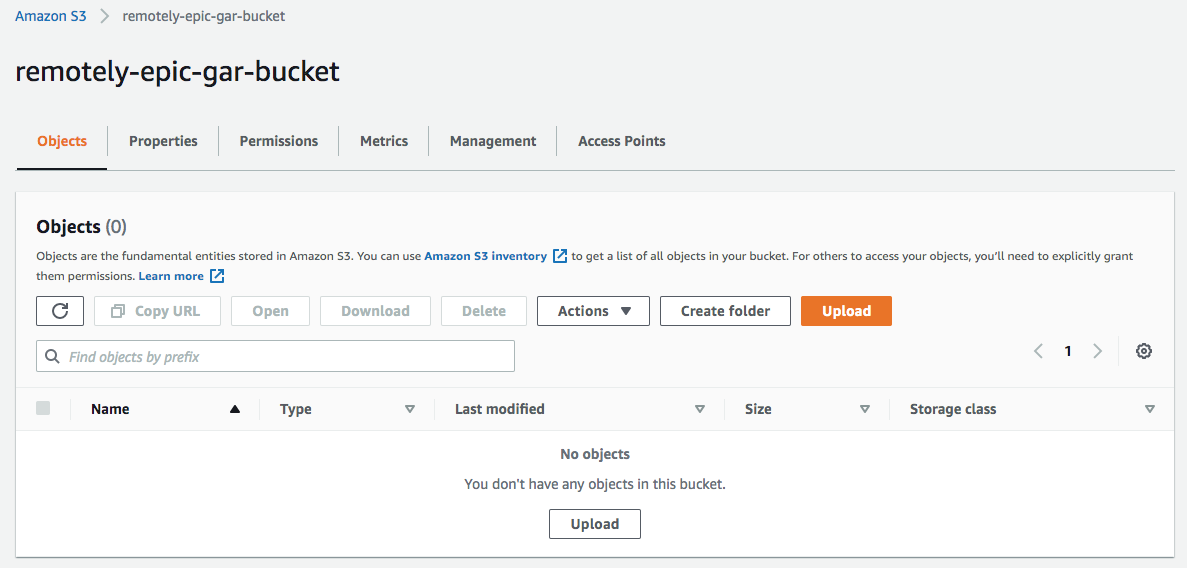
Group policy:
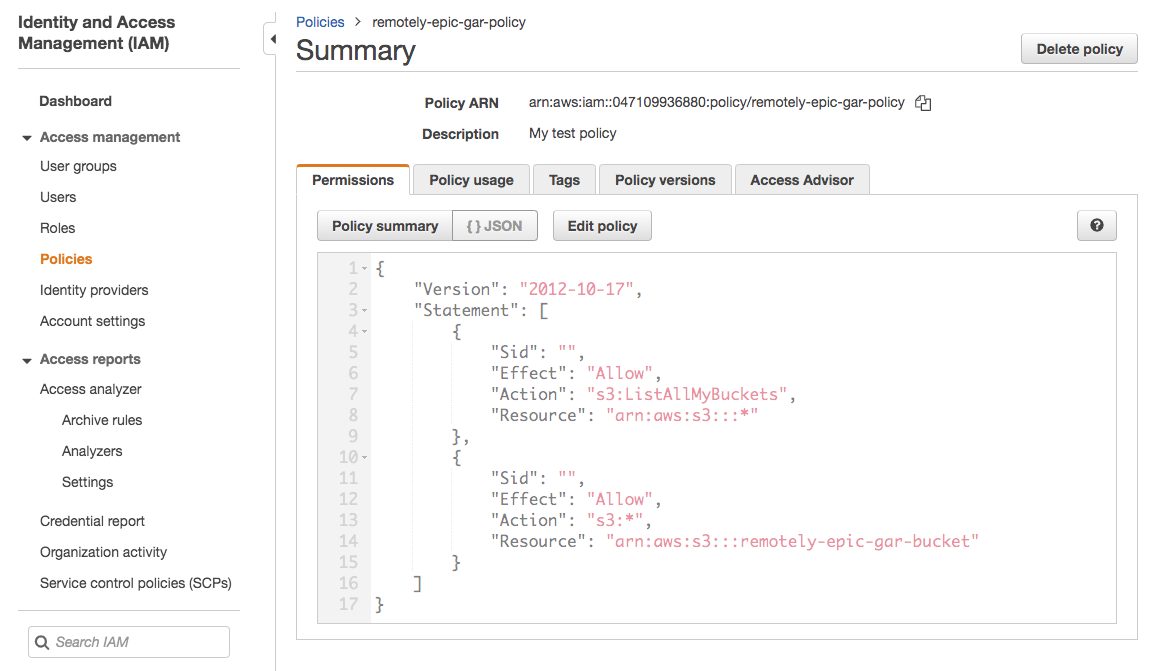
Terraform
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization