13. Django 1.8 Server Build - CentOS 7 hosted on VPS (image/video uploading via ImageField/FileField)
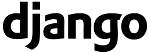
The files that are uploaded by the user such as images, pdfs, videos etc. are our media files. In this tutorial, we will learn how to update database, save, and retrieve the files, specially, images.
In this tutorial, we'll discuss two samples of image uploading (one just for tutorial's sake and the other one for use case of production level). These two examples uses models.ImageField().
We may want to be able to handle video files as well. For video file, we use models.FileField(). This case is handled in later section of this tutorial.
Let's do a sql dump for our car, and it will show all tables so far:
$ python manage.py sqlall car BEGIN; CREATE TABLE `car_car` ( `id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `name` varchar(200) NOT NULL, `slug` varchar(50) NOT NULL UNIQUE, `make_id` integer NOT NULL, `locality` varchar(1) NOT NULL, `description` longtext NOT NULL ) ; CREATE TABLE `car_make` ( `id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `name` varchar(200) NOT NULL, `slug` varchar(50) NOT NULL UNIQUE, `description` longtext NOT NULL ) ; ALTER TABLE `car_car` ADD CONSTRAINT `make_id_refs_id_cde049aa` FOREIGN KEY (`make_id`) REFERENCES `car_make` (`id`); CREATE INDEX `car_car_dff6f6ab` ON `car_car` (`make_id`); COMMIT;
Now, we want to add one more row (ImageField) to car table in models.py:
from django.db import models CAR_CHOICES = ( ('D','Domestic'), ('I','Import'), ) class Car(models.Model): name = models.CharField(max_length=200) slug = models.SlugField(unique=True) make = models.ForeignKey('Make') locality = models.CharField(max_length=1, choices=CAR_CHOICES) description = models.TextField(blank=True) image1 = models.ImageField(upload_to="images/carthumbs/") def __unicode__(self): return self.name class Make(models.Model): name = models.CharField(max_length=200) slug = models.SlugField(unique=True) description = models.TextField(blank=True) def __unicode__(self): return self.name
In the code, the ImageField has been added to our model, defining the upload_to option to specify a subdirectory of MEDIA_ROOT to use for uploaded files.
Before we use the ImageField, we need to install pillow:
$ sudo yum install python-devel $ sudo pip install pillow
Try to update db, manage.py syncdb:
$ python manage.py syncdb Operations to perform: Synchronize unmigrated apps: staticfiles, car, tinymce, messages, pages Apply all migrations: admin, contenttypes, auth, sessions Synchronizing apps without migrations: Creating tables... Running deferred SQL... Installing custom SQL... Running migrations: No migrations to apply.
However, when we look into the table, no table update!
$ ssh -L 8080:localhost:80 -l sfvue 45.79.90.218
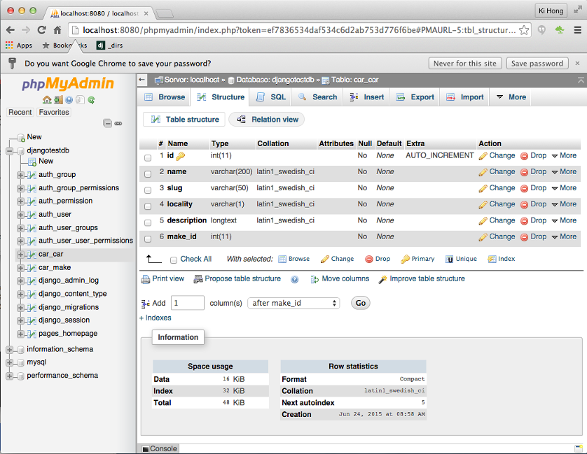
Strangely, the sqlall shows a new field:
$ python manage.py sqlall car BEGIN; CREATE TABLE `car_car` ( `id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `name` varchar(200) NOT NULL, `slug` varchar(50) NOT NULL UNIQUE, `make_id` integer NOT NULL, `locality` varchar(1) NOT NULL, `description` longtext NOT NULL, `image1` varchar(100) NOT NULL ) ; CREATE TABLE `car_make` ( `id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `name` varchar(200) NOT NULL, `slug` varchar(50) NOT NULL UNIQUE, `description` longtext NOT NULL ) ; ALTER TABLE `car_car` ADD CONSTRAINT `make_id_refs_id_cde049aa` FOREIGN KEY (`make_id`) REFERENCES `car_make` (`id`); CREATE INDEX `car_car_dff6f6ab` ON `car_car` (`make_id`); COMMIT;
This is because manage.py will not modify the database schema to match models. It only checks to see if the tables been created. So, if we modified the model after the tables been created, we have to go in and do changes ourselves. Of course, we do not want to drop the table and create it again using syncdb because we will lose laready existing data.
So, let's use phpMyAdmin, and click Go button:

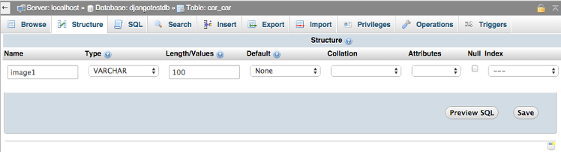
Click "Save", then we can see the new field we've just created:
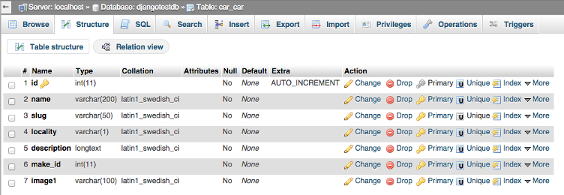
Let's make images/carthumbs and give permissions for the user can upload files:
$ pwd /srv/www/djangotest.sfvue.com/public_html [sfvue@sf public_html]$ mkdir -p media/images/carthumbs [sfvue@sf public_html]$ chmod -R 777 media
Also, we need MEDIA_URL and MEDIA_ROOT in settings.py similar to STATIC_URL and STATIC_ROOT:
STATIC_URL = '/static/' STATIC_ROOT = '/srv/www/djangotest.sfvue.com/public_html/static' MEDIA_URL = '/media/' MEDIA_ROOT = '/srv/www/djangotest.sfvue.com/public_html/media'
Restart our web server:
$ sudo apachectl restart
Go to Admin page:
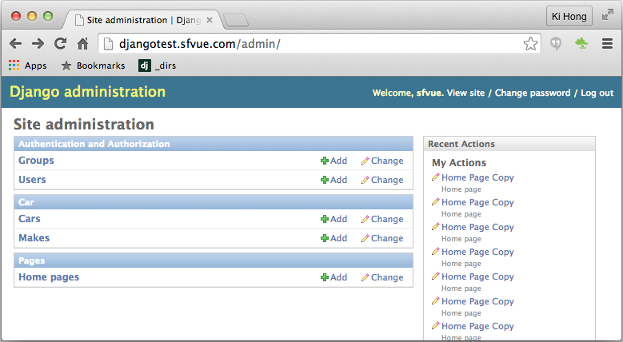
Select "Cars":
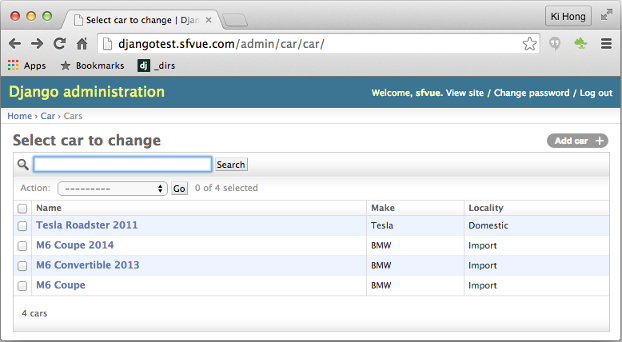
Click a car:
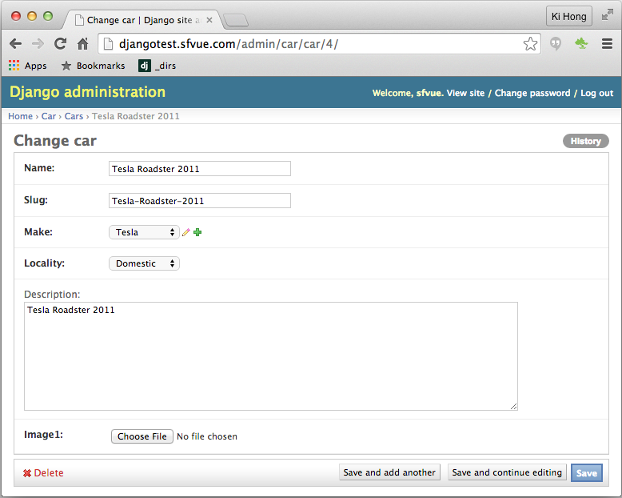
As we can see, we now have an ImageField at the bottom of the page.
Let's try add a new car with its image:
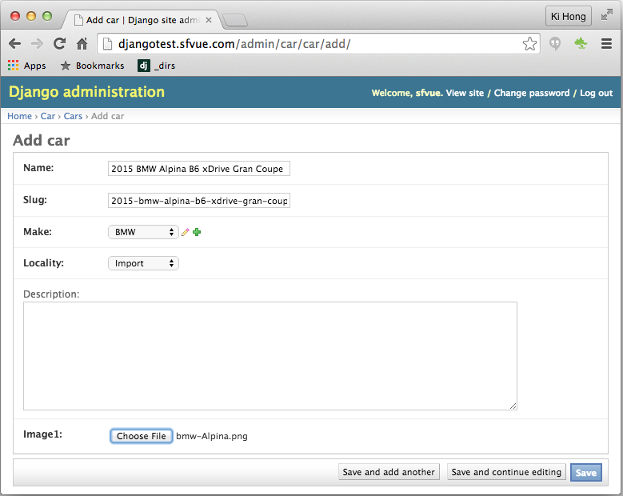
Hit "Save" button:
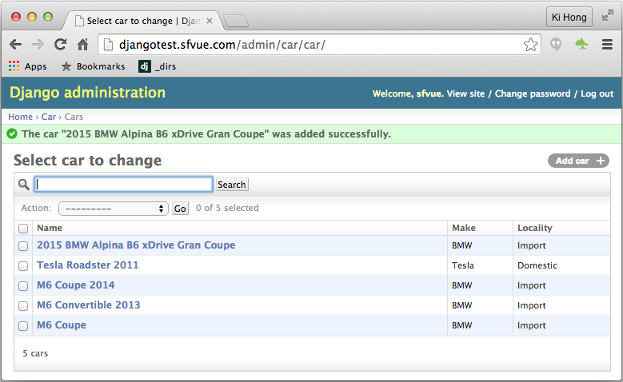
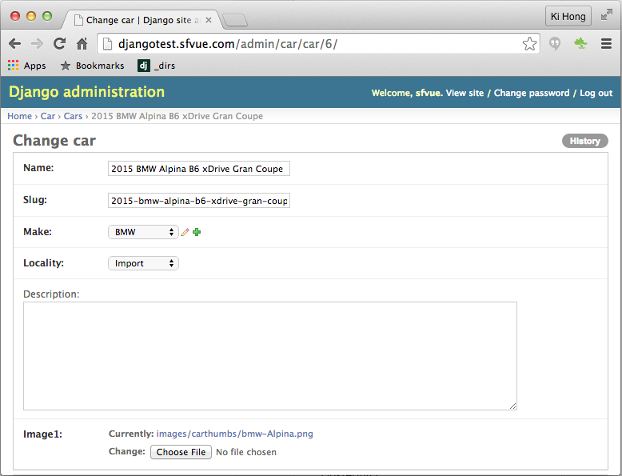
Here is the phpAdmin, after all the images to each car:
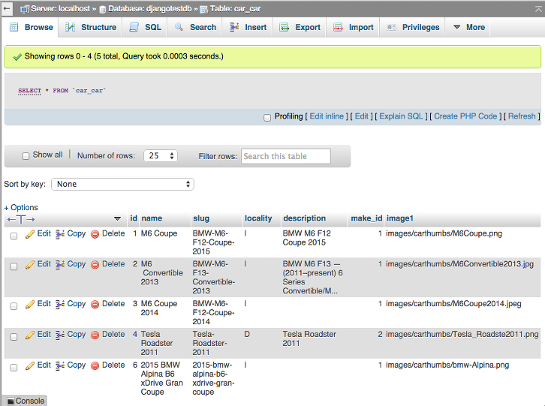
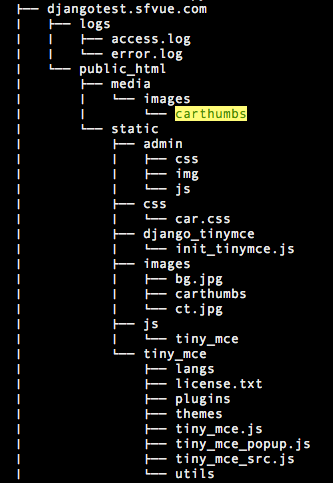
We can serve user-uploaded media files from MEDIA_ROOT using the django.contrib.staticfiles.views.serve() view. This is not suitable for production use!
If the MEDIA_URL is defined as /media/, we can do this by adding the following snippet to our urls.py:
urls.py:
from django.conf.urls import include, url from django.contrib import admin from django.conf import settings urlpatterns = [ url(r'^admin/', include(admin.site.urls)), url(r'^tinymce/', include('tinymce.urls')), url(r'^$', 'pages.views.MainHomePage'), url(r'^cars/$', 'car.views.CarsAll'), url(r'^cars/(?P<carslug>.*)/$', 'car.views.SpecificCar'), url(r'^makes/(?P<makeslug>.*)/$', 'car.views.SpecificMake'), url(r'^media/(?P<path>.*)$', 'django.views.static.serve', { 'document_root': settings.MEDIA_ROOT, }), ]
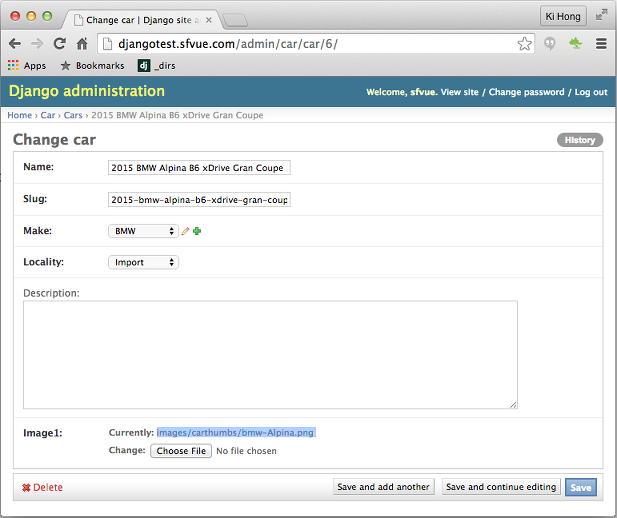
If we click the link for the current image, we get:
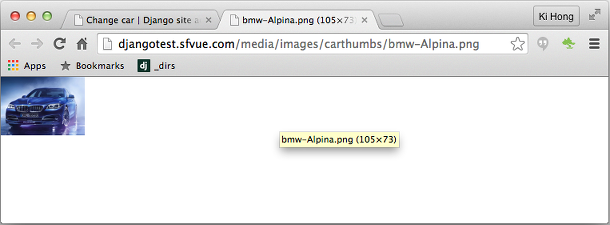
Finally, we get the list of cars with images:
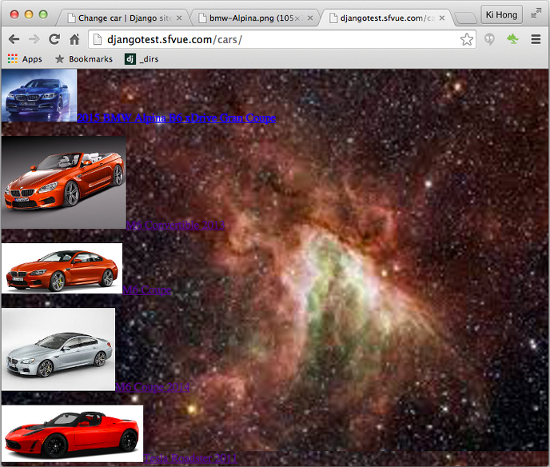
Full source code is available at https://github.com/Einsteinish/Einstein.git.
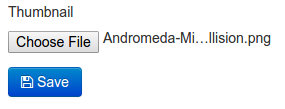
resources/models.py:
from django.db import models from django.contrib.auth.models import User from djangoratings.fields import RatingField from django.core.urlresolvers import reverse from sfvue.utils import unique_slugify from django.template.defaultfilters import slugify LEVELS = ['introductory', 'intermediate', 'in-depth'] class Topic(models.Model): name = models.CharField(max_length=60, unique=True) slug = models.SlugField(max_length=255) help_text = models.CharField(max_length=255, null=True, blank=True) description = models.TextField(null=True, blank=True) thumbnail = models.ImageField(upload_to='topics/%Y/%m/%d/', null=True, blank=True) official_website = models.URLField(null=True, blank=True) class Meta: ordering = ['name',] def __unicode__(self): return self.name def get_absolute_url(self): return reverse('resource_topic_home', kwargs={'slug': self.slug}) def save(self, *args, **kwargs): if not self.slug: self.slug = slugify(self.name) if self.description and not self.help_text: self.help_text = self.description.replace("\n", " ")[:220] super(Topic, self).save(*args, **kwargs) class ResourceType(models.Model): name = models.CharField(max_length=60, unique=True) slug = models.SlugField(max_length=255) help_text = models.CharField(max_length=255, null=True, blank=True) color = models.CharField(max_length=20, default='purple', unique=True) def __unicode__(self): return self.name def get_absolute_url(self): return reverse('resource_list', kwargs={'slug': self.slug}) def save(self, *args, **kwargs): if not self.slug: self.slug = slugify(self.name) super(ResourceType, self).save(*args, **kwargs) class Resource(models.Model): title = models.CharField(max_length=255, unique=True) slug = models.SlugField(max_length=255, blank=True, default='') url = models.URLField(unique=True) help_text = models.CharField(max_length=255, null=True, blank=True) description = models.TextField(null=True, blank=True, default='') DEFAULT_RESOURCE_TYPE_ID=2 resource_type = models.ForeignKey(ResourceType, default=DEFAULT_RESOURCE_TYPE_ID) CHOICES=zip(LEVELS,LEVELS) level = models.CharField('Level of depth', max_length=30, choices=CHOICES, blank=True, default=CHOICES[0][0]) topics = models.ManyToManyField(Topic) created_by = models.ForeignKey(User) rating = RatingField(range=5, weight=10, use_cookies=True, allow_delete=True) created_at = models.DateTimeField(auto_now_add=True, editable=False) updated_at = models.DateTimeField(auto_now=True, editable=False) show = models.BooleanField(default=True) thumbnail = models.ImageField(upload_to='resources/%Y/%m/%d/', null=True, blank=True) def __unicode__(self): return self.title def get_absolute_url(self): return reverse('resource_detail', kwargs={'pk': self.id}) def save(self, *args, **kwargs): #INFO Checks if present because admins have option to change slug if not self.slug: slug_str = '%s' % self.title unique_slugify(self, slug_str) if self.description and not self.help_text: self.help_text = self.description.replace("\n", " ")[:220] super(Resource, self).save(*args, **kwargs) def check_featured(self): for topic in self.topics.all(): try: fr = FeaturedResource.objects.get(resource=self, topic=topic, resource_type=self.resource_type) return True except FeaturedResource.DoesNotExist: pass return False def make_featured(self, topic=None): if self.topics.count()==1: t = self.topics.all()[0] elif topic: if topic in self.topics.all(): t = topic else: return False try: fr = FeaturedResource.objects.get(topic=t, resource_type=self.resource_type) fr.resource = self fr.save() except FeaturedResource.DoesNotExist: fr = FeaturedResource.objects.create(topic=t, resource_type=self.resource_type, resource=self) return True class FeaturedResource(models.Model): topic = models.ForeignKey(Topic) resource_type = models.ForeignKey(ResourceType) resource = models.ForeignKey(Resource) class Meta: unique_together = ('topic', 'resource_type') def __unicode__(self): return '%s - %s' %(self.topic, self.resource_type) def clean(self): from django.core.exceptions import ValidationError if self.resource_type != self.resource.resource_type: raise ValidationError("Selected resource type does not match with given resource's type.") if not self.topic in self.resource.topics.all(): raise ValidationError("Selected resource does not have given topic.") from guardian.shortcuts import assign_perm from django.db.models.signals import post_save def create_resource_permission(sender, instance, created, **kwargs): if created: assign_perm('change_resource', instance.created_by, instance) assign_perm('delete_resource', instance.created_by, instance) post_save.connect(create_resource_permission, sender=Resource)
templates/resources/resource_home.html:
{% extends "resources/base.html" %} {% block head_title %}Resources - Select a topic to start discussion{% endblock %} {% block extra_head %} <link rel="alternate" type="application/atom+xml" title="Recent Resources-Rss" href="{% url 'resource_feed_rss' %}" /> <link rel="alternate" type="application/atom+xml" title="Recent Resources-Atom" href="{% url 'resource_feed_atom' %}" /> {% endblock %} {% block resource_page %} <div class="row-fluid"> <div class="span12"> <div class="span7"> <h3>Select a topic to start discussion</h3> <hr /> </div> <div class="span5"> <a href="{% url 'resource_create' %}" id="resource-add-button" class="btn btn-danger pull-right" title="Add new resource that you want to share" ><i class="icon-plus"></i> Add new Resource</a> </div> </div> <div class="span12 explore-box"> {% for topic in topics %} <article class="span2 topic"> <a title="{{ topic.help_text|slice:":120" }}" href="{{ topic.get_absolute_url }}"> <div class="topic-name"> <span>{{ topic.name }}</span> </div> <div class="topic-image"> {% if topic.thumbnail %} <img src="{{ topic.thumbnail.url }}" alt="{{ topic.name }}" /> {% else %} <img src="{{ STATIC_URL }}img/topic.png" alt="{{ topic.name }} image" /> {% endif %} </div> </a> </article> {% endfor %} </div> </div> <div class="row-fluid"> <p class="muted disclaimer">If you don't see the topic, please create a new one.</p> <p>Subscribe for Updates: <a class="btn btn-small" href="{% url 'resource_feed_rss' %}"><i class="icon-rss"></i> Rss</a> <a class="btn btn-small" href="{% url 'resource_feed_atom' %}"><i class="icon-rss-sign"></i> Atom</a></p> </div> {% endblock %}
templates/resources/resource_form.html:
{% extends "resources/base.html" %} {% block resource_content %} <p> <a href="{% if request.META.HTTP_REFERER %}{{ request.META.HTTP_REFERER }}{% else %}{% url 'resource_home' %}{% endif %}" class="btn"><i class="icon-double-angle-left"></i> Back</a> </p> <div class="span8 offset2"> <form action="" method="post" enctype="multipart/form-data"> {% include "snippets/forms/form_base_block.html" %} <button class="btn btn-primary" type="submit"><i class="icon-save"></i> Save</button> </form> </div> {% endblock %}
In this section, we'll briefly discuss video upload in Django.
Here is the screen shot from one of the pages of http://einsteinish.com/:
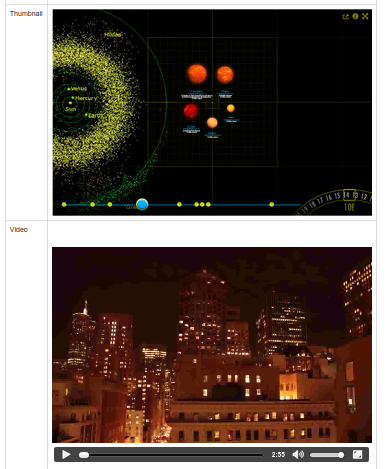
As we can see we have two items: image and video. They are actually uploaded from the following form:
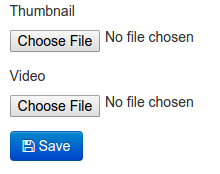
templates/resources/resource_form.html:
{% extends "resources/base.html" %} {% block resource_content %} <p> <a href="{% if request.META.HTTP_REFERER %}{{ request.META.HTTP_REFERER }}{% else %}{% url 'resource_home' %}{% endif %}" class="btn"><i class="icon-double-angle-left"></i> Back</a> </p> <div class="span8 offset2"> <form action="" method="post" enctype="multipart/form-data"> {% include "snippets/forms/form_base_block.html" %} <button class="btn btn-primary" type="submit"><i class="icon-save"></i> Save</button> </form> </div> {% endblock %}
templates/resources/snippets/forms/form_base_block.html:
{% load widget_tweaks %} {% csrf_token %} {% if form.non_field_errors %} {% for non_field_error in form.non_field_errors %} <div class="alert alert-error"> <button class="close" type="button" data-dismiss="alert">×</button> {{ non_field_error }} </div> {% endfor %} {% endif %} {% for field in form.hidden_fields %} {{ field }} {% endfor %} {% for field in form.visible_fields %} <div class="control-group{% if field.errors %} error{% endif %}"> <label for="{{ field.auto_id }}" class="control-label">{{ field.label }}{% if field.field.required %}*{% endif %} </label> <div class="controls"> {{ field|add_class:"input-block-level" }} {% if field.errors %} {% for error in field.errors %} <span class="help-inline field-error">{{ error }}</span> {% endfor %} {% endif %} {% if field.help_text %}<span class="help-block"><em>{{ field.help_text|safe }}</em></span>{% endif %} </div> </div> {% endfor %}
resources/models.py:
from django.db import models from django.contrib.auth.models import User from djangoratings.fields import RatingField from django.core.urlresolvers import reverse from einsteinish.utils import unique_slugify from django.template.defaultfilters import slugify LEVELS = ['introductory', 'intermediate', 'in-depth'] class Topic(models.Model): name = models.CharField(max_length=60, unique=True) slug = models.SlugField(max_length=255) help_text = models.CharField(max_length=255, null=True, blank=True) description = models.TextField(null=True, blank=True) thumbnail = models.ImageField(upload_to='topics/%Y/%m/%d/', null=True, blank=True) official_website = models.URLField(null=True, blank=True) class Meta: ordering = ['name',] def __unicode__(self): return self.name def get_absolute_url(self): return reverse('resource_topic_home', kwargs={'slug': self.slug}) def save(self, *args, **kwargs): if not self.slug: self.slug = slugify(self.name) if self.description and not self.help_text: self.help_text = self.description.replace("\n", " ")[:220] super(Topic, self).save(*args, **kwargs) class ResourceType(models.Model): name = models.CharField(max_length=60, unique=True) slug = models.SlugField(max_length=255) help_text = models.CharField(max_length=255, null=True, blank=True) color = models.CharField(max_length=20, default='purple', unique=True) def __unicode__(self): return self.name def get_absolute_url(self): return reverse('resource_list', kwargs={'slug': self.slug}) def save(self, *args, **kwargs): if not self.slug: self.slug = slugify(self.name) super(ResourceType, self).save(*args, **kwargs) class Resource(models.Model): title = models.CharField(max_length=255, unique=True) slug = models.SlugField(max_length=255, blank=True, default='') url = models.URLField(unique=True) help_text = models.CharField(max_length=255, null=True, blank=True) description = models.TextField(null=True, blank=True, default='') DEFAULT_RESOURCE_TYPE_ID=2 resource_type = models.ForeignKey(ResourceType, default=DEFAULT_RESOURCE_TYPE_ID) CHOICES=zip(LEVELS,LEVELS) level = models.CharField('Level of depth', max_length=30, choices=CHOICES, blank=True, default=CHOICES[0][0]) topics = models.ManyToManyField(Topic) created_by = models.ForeignKey(User) rating = RatingField(range=5, weight=10, use_cookies=True, allow_delete=True) created_at = models.DateTimeField(auto_now_add=True, editable=False) updated_at = models.DateTimeField(auto_now=True, editable=False) show = models.BooleanField(default=True) # new thumbnail = models.ImageField(upload_to='resources/%Y/%m/%d/', null=True, blank=True) video = models.FileField(upload_to='resources/%Y/%m/%d/', null=True, blank=True) def __unicode__(self): return self.title def get_absolute_url(self): return reverse('resource_detail', kwargs={'pk': self.id}) def save(self, *args, **kwargs): #INFO Checks if present because admins have option to change slug if not self.slug: slug_str = '%s' % self.title unique_slugify(self, slug_str) if self.description and not self.help_text: self.help_text = self.description.replace("\n", " ")[:220] super(Resource, self).save(*args, **kwargs) def check_featured(self): for topic in self.topics.all(): try: fr = FeaturedResource.objects.get(resource=self, topic=topic, resource_type=self.resource_type) return True except FeaturedResource.DoesNotExist: pass return False def make_featured(self, topic=None): if self.topics.count()==1: t = self.topics.all()[0] elif topic: if topic in self.topics.all(): t = topic else: return False try: fr = FeaturedResource.objects.get(topic=t, resource_type=self.resource_type) fr.resource = self fr.save() except FeaturedResource.DoesNotExist: fr = FeaturedResource.objects.create(topic=t, resource_type=self.resource_type, resource=self) return True class FeaturedResource(models.Model): topic = models.ForeignKey(Topic) resource_type = models.ForeignKey(ResourceType) resource = models.ForeignKey(Resource) class Meta: unique_together = ('topic', 'resource_type') def __unicode__(self): return '%s - %s' %(self.topic, self.resource_type) def clean(self): from django.core.exceptions import ValidationError if self.resource_type != self.resource.resource_type: raise ValidationError("Selected resource type does not match with given resource's type.") if not self.topic in self.resource.topics.all(): raise ValidationError("Selected resource does not have given topic.") from guardian.shortcuts import assign_perm from django.db.models.signals import post_save def create_resource_permission(sender, instance, created, **kwargs): if created: assign_perm('change_resource', instance.created_by, instance) assign_perm('delete_resource', instance.created_by, instance) post_save.connect(create_resource_permission, sender=Resource)
The actual video embedding code (templates/resources/resource_detail.html) looks like this:
{% extends "base.html" %} {% load age issaved from einsteinish %} {% load disqus_tags %} {% load markdown_deux_tags %} {% block meta_description %}{{ resource.help_text }} - {{ resource.resource_type }} submitted by {{ resource.created_by }}{% endblock %} {% block content %} <div class="row-fluid"> <div class="container"> {% block resource_page %} <div class="row-fluid"> <div class="span9 resource-content"> <div class="row-fluid well-small" id="resource-title"> <h1><a href="{{ resource.url }}" target="_blank">{{ resource.title }}</a></h1> <hr /> </div> <div class="btn-toolbar" id="resource-buttons"> <div class="btn-group"> <a href="{{ resource.url }}" target="_blank" class="btn btn-small btn-info"><i class="icon-link"></i> Open Webpage</a> </div> {% if user.is_authenticated %} <div class="btn-group"> <a href="{% url 'resource_save' pk=resource.pk %}" title="Save this resource to your profile" class="btn btn-small {% if resource|issaved:user %}disabled{% endif %}"><i class="icon-save"></i> {% if resource|issaved:user %}Saved{% else %}Save{% endif %}</a> {% ifequal resource.created_by user%} <a href="{% url 'resource_update' pk=resource.id %}" class="btn btn-small"><i class="icon-edit"></i> Edit</a> {% endifequal %} </div> {% include "resources/rating.html" %} {% else %} <div class="btn-group"> <a href="{% url 'auth_login' %}?next={% url 'resource_detail' pk=resource.pk %}" class="btn btn-small"><i class="icon-info"></i> Login to rate or save this resource</a> </div> {% endif %} </div> <div class="row-fluid"> {% include "snippets/share_this.html" %} </div> <div class="row-fluid"> <div class="span8 offset2"> <table class="table table-bordered table-stripped"> <tbody> {% with approval=resource.rating.get_real_rating %} <tr class="{% if approval >= 3 %}success{% endif %}"> <td>Rating</td> <td>{{ approval|floatformat }}/5</td> </tr>{% endwith %} <tr> <td>Resource Type</td> <td>{{ resource.resource_type }}</td> </tr> <tr> <td>Topics</td> <td> {% for topic in resource.topics.all %} {% if not forloop.first %}, {% endif %}<a href="{% url 'resource_topic_home' slug=topic.slug %}" class="">{{ topic.name }}</a> {% endfor %} </td> </tr> <tr> <td>Submitted by</td> <td><a href="{{ resource.created_by.get_absolute_url }}">{{ resource.created_by }}</a></td> </tr> <tr> <td>Date Submitted</td> <td>{{ resource.created_at|date:"D d M Y" }}</td> </tr> {% if resource.thumbnail %} <tr> <td>Thumbnail</td> <td> <img src="{{ resource.thumbnail.url }}" alt="{{ resource.name }} logo" class="pull-right" /> </td> </tr> {% endif %} {% if resource.video %} <tr> <td>Video</td> <td> <video width="640" height="480" controls> <source src="{{ resource.video.url }}" type="video/mp4"> <source src="{{ resource.video.url }}" type="video/ogg"> Your browser does not support the video tag. </video> </td> </tr> {% endif %} </tbody> </table> </div> </div> {% if resource.description %} <div class="row-fluid markdown-text" id="resource-description"> {{ resource.description|markdown:"safe" }} </div> {% endif %} <div class="row-fluid"> {% disqus_show_comments %} </div> </div> <div class="span3 well" id="sidebar">{% include "resources/topics.html" %}</div> </div> {% endblock %} </div> </div> {% endblock %}
The source codes are available from repo.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization