6. Django 1.8 Server Build - CentOS 7 hosted on VPS - Model
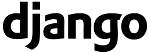
In previous chapter (5. Django 1.8 Server Build - CentOS 7 hosted on VPS - Install and Configure Django), we setup Django project, MySQL database, and Django Admin page (static files).
In this chapter, we'll make an app called car.
Now that our Django project is set up, we're set to start doing real work.
Each application we write in Django consists of a Python package that follows a certain convention. Django comes with a utility that automatically generates the basic directory structure of an app, so we can focus on writing code rather than creating directories.
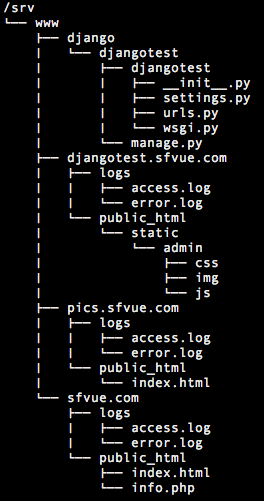
As we already know, a project is a collection of configurations and apps for a particular Web site. A project can contain multiple apps. An app can be in multiple projects. Our apps can live anywhere on our Python path.
In this tutorial, we'll create our car app right next to our manage.py file so that it can be imported as its own top-level module, rather than a sub-module of a site.
To create our app, we need to make sure we're in the same directory as manage.py and type the following command:
[sfvue@sf djangotest]$ python manage.py startapp car
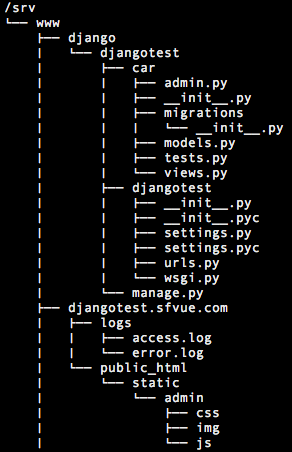
As we can see, car folder has been created by manage.py:
[sfvue@sf djangotest]$ ls car djangotest manage.py
Let's go into the car directory:
$ cd car admin.py __init__.py migrations models.py tests.py views.py
In this chapter, we'll mainly work on models.py.
The first step in writing a database Web app in Django is to define our models - essentially, our database layout, with additional metadata, and here is our model:
Car name make locality (domestic or foreign) description Make name description
The models.py looks like this:
from django.db import models CAR_CHOICES = ( ('D','Domestic'), ('I','Import'), ) class Car(models.Model): name = models.CharField(max_length=200) slug = models.SlugField(unique=True) make = models.ForeignKey('Make') locality = models.CharField(max_length=1, choices=CAR_CHOICES) description = models.TextField(blank=True) def __unicode__(self): return self.name class Make(models.Model): name = models.CharField(max_length=200) slug = models.SlugField(unique=True) description = models.TextField(blank=True) def __unicode__(self): return self.name
The slug is a newspaper term. A slug is a short label for something, containing only letters, numbers, underscores or hyphens. They're generally used in URLs.
Like a CharField, we can specify max_length (read the note about database portability and max_length in that section, too). If max_length is not specified, Django will use a default length of 50.As a side note, the method __unicode__() is a preferred method over __str__() method since __unicode__() returns characters while __str__() returns byte. Usually, we should put all our string formatting in __unicode__(), and create a stub __str__() method:
def __str__(self): return unicode(self).encode('utf-8')
Also, we need to add our new app (car) in settings.py:
INSTALLED_APPS = ( 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'car', )
To reflect the changes, we need to use syncdb:
[sfvue@sf djangotest]$ ls car djangotest manage.py [sfvue@sf djangotest]$ python manage.py syncdb /usr/lib64/python2.7/site-packages/django/core/management/commands/syncdb.py:24: RemovedInDjango19Warning: The syncdb command will be removed in Django 1.9 warnings.warn("The syncdb command will be removed in Django 1.9", RemovedInDjango19Warning) Operations to perform: Synchronize unmigrated apps: staticfiles, messages Apply all migrations: admin, contenttypes, auth, sessions Synchronizing apps without migrations: Creating tables... Running deferred SQL... Installing custom SQL... Running migrations: No migrations to apply. Your models have changes that are not yet reflected in a migration, and so won't be applied. Run 'manage.py makemigrations' to make new migrations, and then re-run 'manage.py migrate' to apply them. [sfvue@sf djangotest]$ python manage.py makemigrations Migrations for 'car': 0001_initial.py: - Create model Car - Create model Make - Add field make to car [sfvue@sf djangotest]$ python manage.py migrate Operations to perform: Synchronize unmigrated apps: staticfiles, messages Apply all migrations: admin, car, contenttypes, auth, sessions Synchronizing apps without migrations: Creating tables... Running deferred SQL... Installing custom SQL... Running migrations: Rendering model states... DONE Applying car.0001_initial... OK
To get a better idea what's been done:
[sfvue@sf djangotest]$ python manage.py sqlall car CommandError: App 'car' has migrations. Only the sqlmigrate and sqlflush commands can be used when an app has migrations. [sfvue@sf djangotest]$ ls car djangotest manage.py [sfvue@sf djangotest]$ cd car [sfvue@sf car]$ ls admin.py __init__.py migrations models.pyc views.py admin.pyc __init__.pyc models.py tests.py [sfvue@sf car]$ mv migrations migrations_moved [sfvue@sf car]$ cd .. [sfvue@sf djangotest]$ ls car djangotest manage.py [sfvue@sf djangotest]$ python manage.py sqlall car BEGIN; CREATE TABLE `car_car` ( `id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `name` varchar(200) NOT NULL, `slug` varchar(50) NOT NULL UNIQUE, `make_id` integer NOT NULL, `locality` varchar(1) NOT NULL, `description` longtext NOT NULL ) ; CREATE TABLE `car_make` ( `id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `name` varchar(200) NOT NULL, `slug` varchar(50) NOT NULL UNIQUE, `description` longtext NOT NULL ) ; ALTER TABLE `car_car` ADD CONSTRAINT `make_id_refs_id_cde049aa` FOREIGN KEY (`make_id`) REFERENCES `car_make` (`id`); CREATE INDEX `car_car_dff6f6ab` ON `car_car` (`make_id`); COMMIT; [sfvue@sf djangotest]$
Note here I renamed migrations folder. (see Django CommandError: App 'polls' has migrations)
At this point, when we look at the Django admin page, nothing's been changed. That's because we haven't told the new things to Django admin yet:
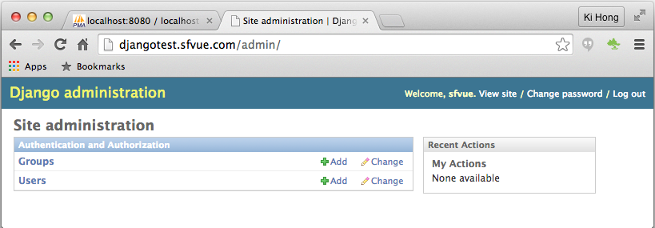
Let's edit /srv/www/django/djangotest/car/admin.py:
from django.contrib import admin from car.models import Car, Make admin.site.register(Car) admin.site.register(Make)
Then, restart the server:
$ sudo apachectl restart
Now, if we refresh the Django admin page, we have a new car app, and inside the app, we have two objects: Cars and Makes:
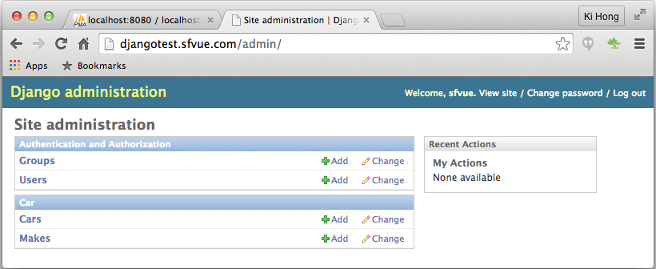
However, if we go to Cars, nothing is there since we haven't populated the table yet:
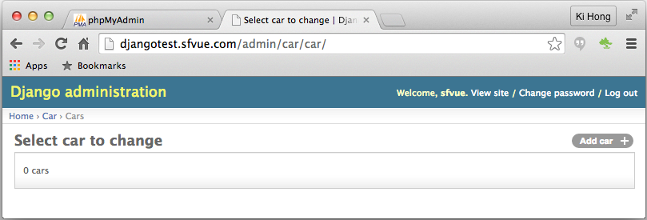
In next chapter (7. Model 2 : populate tables, list_display, and search_fields), we'll populate the tables.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization