Authentication (User Registration A) on Shared Host using FastCGI
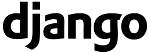
As a continuation from the previous chapter, Authentication on Shared Host using FastCGI. in this chapter, we will implement user registration. We'll need two things: username and password.
We need put two files in ~/www/dj directory:
# pwd /home2/bogotob1/public_html/dj # ls ./ ../ djangoproject.fcgi* .htaccess
djangoproject.fcgi:
#! /home2/bogotob1/python/bin/python import sys, os sys.path.insert(0, "/home2/bogotob1/python") sys.path.insert(13, "/home2/bogotob1/djangoproject") os.environ['DJANGO_SETTINGS_MODULE'] = 'djangoproject.settings' from django.core.servers.fastcgi import runfastcgi runfastcgi(method="threaded", daemonize="false")
where /home2/bogotob1/ is my home(~) directory.
.htaccess:
# django AddHandler fcgid-script .fcgi RewriteEngine On RewriteCond %{REQUEST_FILENAME} !-f RewriteRule ^(.*)$ djangoproject.fcgi/$1 [QSA,L]
To register a user, we're adding two views to the urls previously defined in urls.py:
from django.conf.urls import patterns, include, url from django.contrib import admin admin.autodiscover() urlpatterns = patterns('', # Examples: url(r'^$', 'djangoapp.views.home', name='home'), # url(r'^blog/', include('blog.urls')), url(r'^admin/', include(admin.site.urls)), # user authentication urls url(r'^accounts/login/$', 'djangoapp.views.login'), url(r'^accounts/auth/$', 'djangoapp.views.auth_view'), url(r'^accounts/login/accounts/auth/$', 'djangoapp.views.auth_view'), url(r'^accounts/logout/$', 'djangoapp.views.logout'), url(r'^accounts/loggedin/$', 'djangoapp.views.loggedin'), url(r'^accounts/invalid/$', 'djangoapp.views.invalid_login'), url(r'^accounts/register/$', 'djangoapp.views.register_user'), url(r'^accounts/register_success/$', 'djangoapp.views.register_success'), )
Similar to the urls, we're going to add new views for registration to views.py:
from django.shortcuts import render_to_response from django.http import HttpResponseRedirect from django.contrib import auth from django.core.context_processors import csrf from django.contrib.auth.forms import UserCreationForm def home(request): return render_to_response('home.html', {'Testing' : 'Django Template Inheritance ', 'HelloHello' : 'Hello World - Django'}) def login(request): c = {} c.update(csrf(request)) return render_to_response('login.html', c) def auth_view(request): username = request.POST.get('username', '') password = request.POST.get('password', '') user = auth.authenticate(username=username, password=password) if user is not None: auth.login(request, user) return HttpResponseRedirect('/dj/accounts/loggedin') else: return HttpResponseRedirect('/dj/accounts/invalid') def logout(request): auth.logout(request) return render_to_response('logout.html') def loggedin(request): return render_to_response('loggedin.html', {'full_name': request.user.username}) def invalid_login(request): return render_to_response('invalid_login.html') def register_user(request): # 2nd time around if request.method == 'POST': form = UserCreationForm(request.POST) if form.is_valid(): form.save() return HttpResponseRedirect('/dj/accounts/register_success') # 1st time visit args = {} args.update(csrf(request)) # form with no input args['form'] = UserCreationForm() print args return render_to_response('register.html', args) def register_success(request): return render_to_response('register_success.html')
register.html:
{% extends "base.html" %} {% block content %} <h1>Register</h2> <form action="/dj/accounts/register/" method="post">{% csrf_token %} {{ form }} <input type="Submit" value="Register" /> </form> {% endblock %}
register_success.html:
{% extends "base.html" %} {% block content %} <h2>You are now registered!</h2> <p>Click <a href="/dj/accounts/login/">here</a> to login</p> {% endblock %}
Let's register with username as register_test:
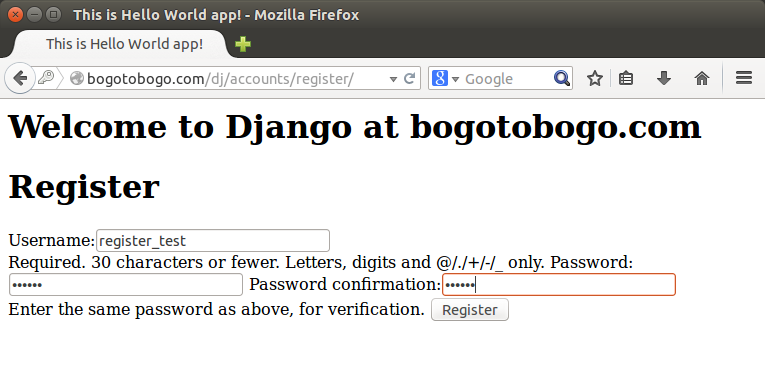
The form from UserCreationForm() is not well aligned, and we'll fix it later. Right now, we just want to check if it's working properly.
If the registration is successful, we get the following page:
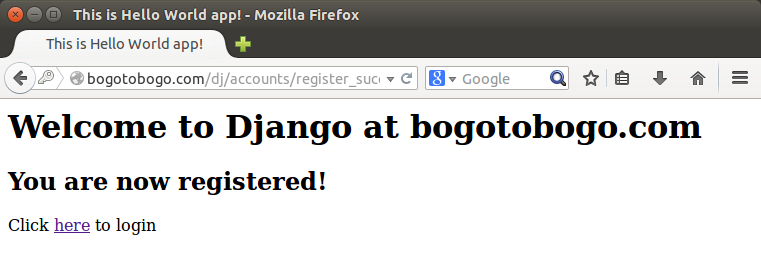
Now, let's login with the newly registered username:
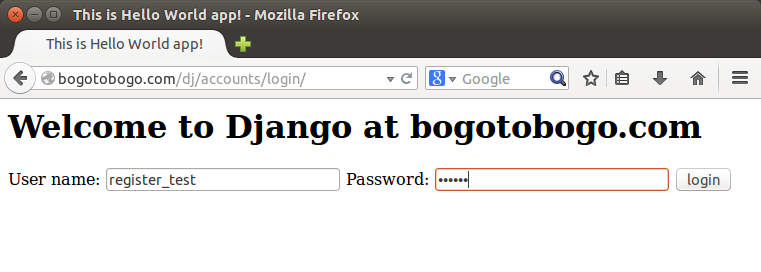
We can see it works:
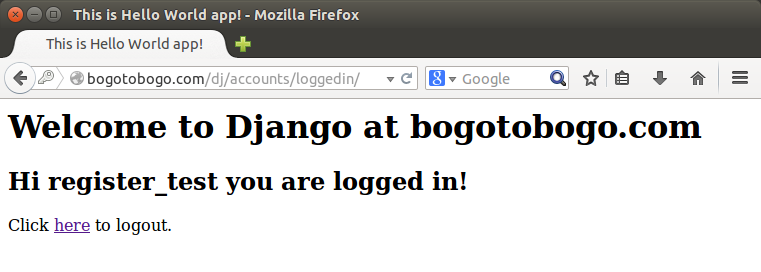
We can check if the user (register_test) is there from admin page:
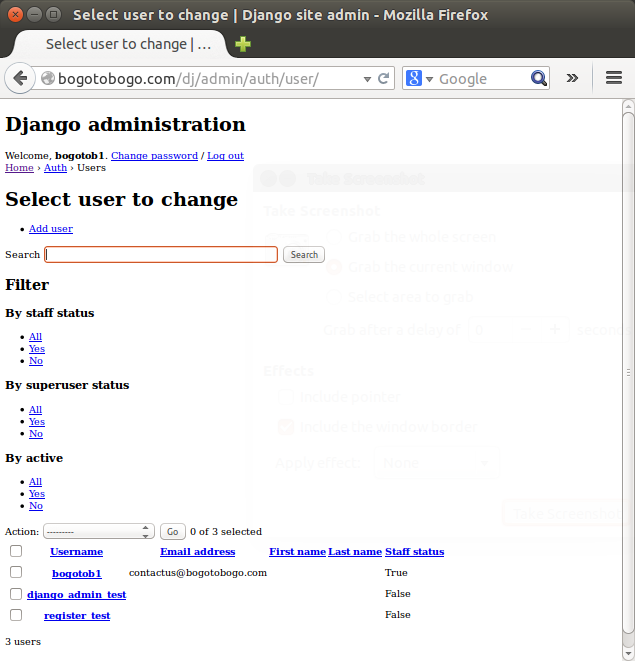
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization