Blog on Shared Host using FastCGI
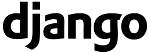
As a continuation from the previous chapters on Shared Host, User Registration on Shared Host using FastCGI. So, it carries some code from the chapter.
In this chapter, we will add blog features as shown in the picture below:
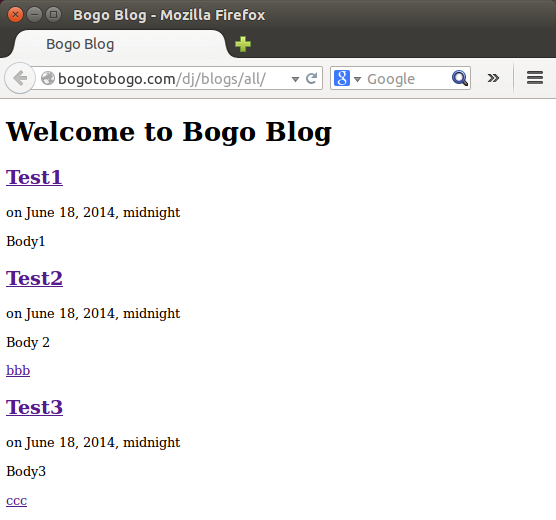
As in the previous chapters, we need keep two files in ~/www/dj directory:
# pwd /home2/bogotob1/public_html/dj # ls ./ ../ djangoproject.fcgi* .htaccess
djangoproject.fcgi:
#! /home2/bogotob1/python/bin/python import sys, os sys.path.insert(0, "/home2/bogotob1/python") sys.path.insert(13, "/home2/bogotob1/djangoproject") os.environ['DJANGO_SETTINGS_MODULE'] = 'djangoproject.settings' from django.core.servers.fastcgi import runfastcgi runfastcgi(method="threaded", daemonize="false")
where /home2/bogotob1/ is my home(~) directory.
.htaccess:
# django AddHandler fcgid-script .fcgi RewriteEngine On RewriteCond %{REQUEST_FILENAME} !-f RewriteRule ^(.*)$ djangoproject.fcgi/$1 [QSA,L]
Let's create an app for blog:
$ cd ~/djangoproject $ python manage.py startapp blog
from django.db import models # Create your models here. class Post(models.Model): title = models.CharField(max_length=200) body = models.TextField() publish_date = models.DateTimeField('published date') # string representation def __unicode__(self): return self.title
First, we need to install taggit:
$ pip install django-taggit Downloading/unpacking django-taggit Downloading django-taggit-0.12.tar.gz Running setup.py egg_info for package django-taggit Installing collected packages: django-taggit Running setup.py install for django-taggit Successfully installed django-taggit Cleaning up...
Add "taggit" to our project's INSTALLED_APPS setting:
INSTALLED_APPS = ( 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'djangoapp', 'taggit', 'blog', )
Run manage.py syncdb:
$ python manage.py syncdb python manage.py syncdb Creating tables ... Creating table taggit_tag Creating table taggit_taggeditem Installing custom SQL ... Installing indexes ... Installed 0 object(s) from 0 fixture(s)
And then do the following to enable tagging on our Post:
from django.db import models from taggit.managers import TaggableManager # Create your models here. class Post(models.Model): title = models.CharField(max_length=200) body = models.TextField() publish_date = models.DateTimeField('published date') tags = TaggableManager() # string representation def __unicode__(self): return self.title
We need to register our Post model via blog/admin.py:
from django.contrib import admin from blog.models import Post # Register your models here. admin.site.register(Post)
Now we can see our Posts and Tags have been included in Admin:
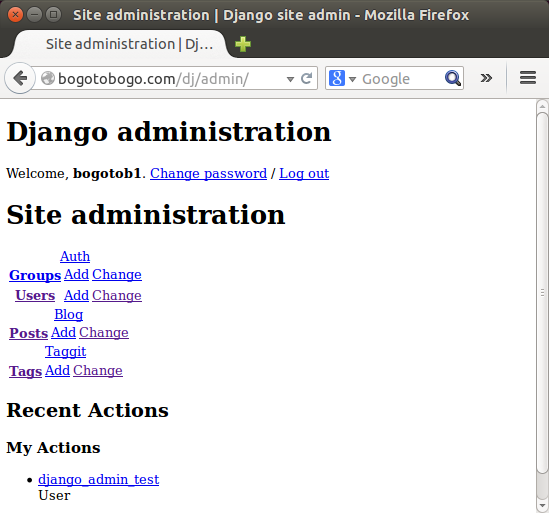
We may want to create couple of posts:
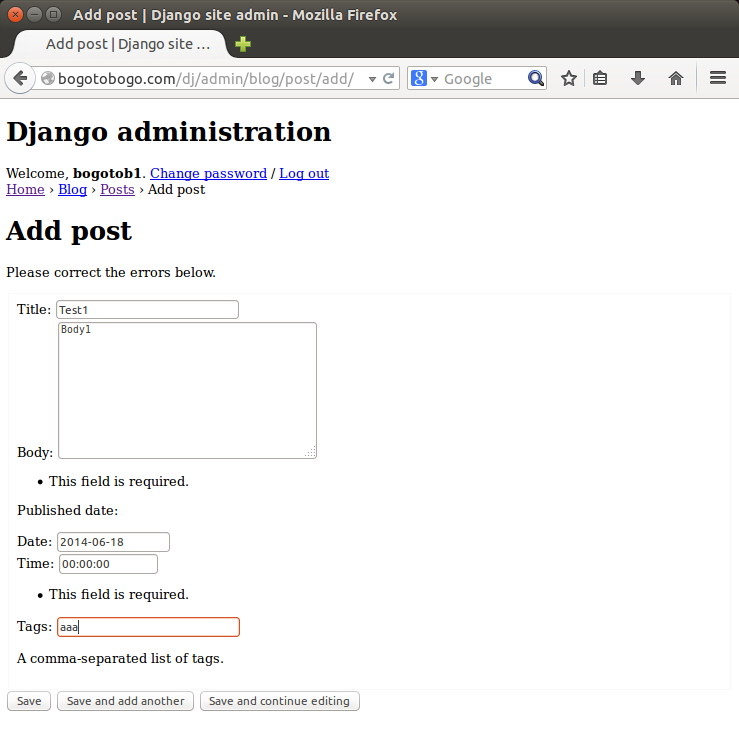
After adding 3 posts:
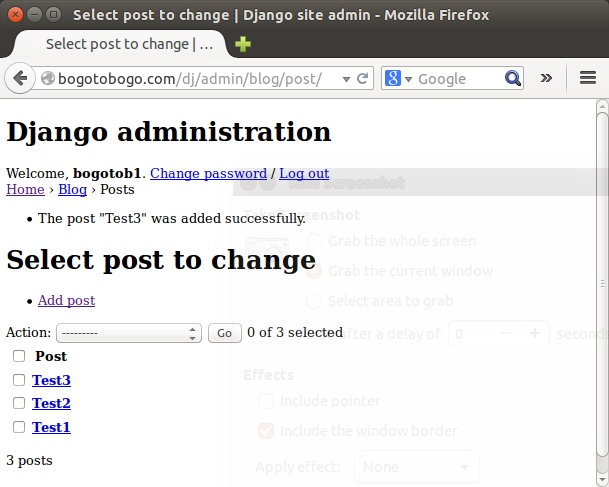
from django.contrib import admin admin.autodiscover() urlpatterns = patterns('', url(r'^$', 'djangoapp.views.home', name='home'), url(r'^admin/', include(admin.site.urls)), # user authentication urls url(r'^accounts/login/$', 'djangoapp.views.login'), url(r'^accounts/auth/$', 'djangoapp.views.auth_view'), url(r'^accounts/login/accounts/auth/$', 'djangoapp.views.auth_view'), url(r'^accounts/logout/$', 'djangoapp.views.logout'), url(r'^accounts/loggedin/$', 'djangoapp.views.loggedin'), url(r'^accounts/invalid/$', 'djangoapp.views.invalid_login'), url(r'^accounts/register/$', 'djangoapp.views.register_user'), url(r'^accounts/register_success/$', 'djangoapp.views.register_success'), # blog url(r'^blogs/', include('blog.urls')), )
from django.conf.urls import patterns, include, url urlpatterns = patterns('', url(r'^all/$', 'blog.views.blogs'), url(r'^get/(?P<post_id>\d+)/$', 'blog.views.blog'), url(r'^tag/(?P<tag>\w+)/$', 'blog.views.tagpage'), )
from django.shortcuts import render_to_response from blog.models import Post def blogs(request): return render_to_response('blogs.html', {'blogs': Post.objects.all() }) # passing post_id to the view def blog(request, post_id = 1): return render_to_response('blog.html', {'post': Post.objects.get(id = post_id) }) def tagpage(request, tag): posts = Post.objects.filter(tags__name = tag) return render_to_response('tagpage.html', {'posts': posts, 'tag':tag })
<!DOCTYPE html> <html> <head> <title>Bogo Blog</title> </head> <h1>Welcome to Bogo Blog </h1> <body> {% block content %}{% endblock %} </body> </html>
{% extends "blog_base.html" %} {% block content %} <div> <h2><a href="/dj/blogs/get/{{post.id}}/">{{ post.title }}</a></h2> </div> <div class="post.meta"> on {{ post.publish_date }} </div> <div class="post_body"> {{ post.body|safe|linebreaks }} </div> <div class="tags"> {% for tag in post.tags.all %} <a href="/dj/blogs/tag/{{tag}}">{{tag}}</a> {% endfor %} </div> {% endblock %}
{% extends "blog_base.html" %} {% block content %} {% for post in blogs %} <div> <h2><a href="/dj/blogs/get/{{post.id}}/">{{ post.title }}</a></h2> </div> <div class="post.meta"> on {{ post.publish_date }} </div> <div class="post_body"> {{ post.body|safe|linebreaks }} </div> <div class="tags"> {% for tag in post.tags.all %} <a href="/dj/blogs/tag/{{tag}}">{{tag}}</a> {% endfor %} </div> {% endfor %} {% endblock %}
{% extends "blog_base.html" %} {% block content %} <h2>Tagged posts: {{tag}}</h2> {% for post in posts %} <p> {{ post.publish_date|date:"Y-m-d" }}: <a href="dj/blog/{{post.id}}">{{post.title}}</a></p> {% endfor %} {% endblock %}
Displaying all the posts:
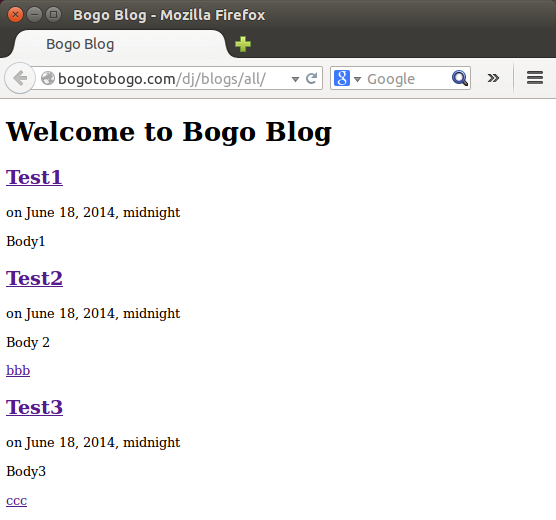
Displaying a specific post:
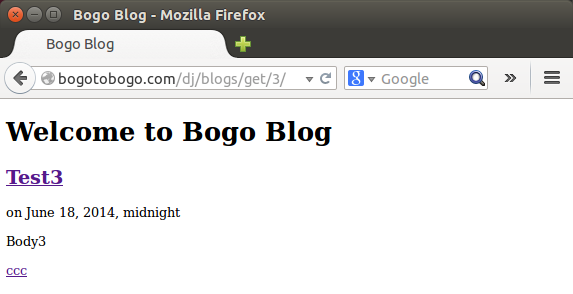
Displaying a tagged post:
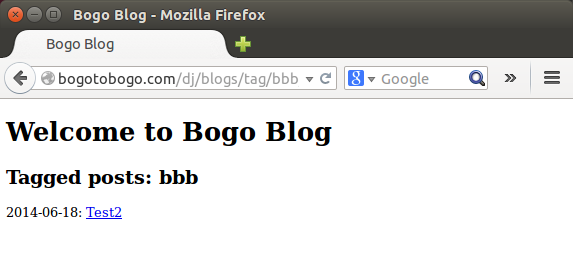
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization