2. Creating and Activating Models
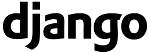
This chapter is a continuation from the Introduction, but we may want to skip this and go to Hello World - Django which has a simpler example, and it'll help us to learn Django more quickly. We can come back to this chapter after that.
With the model we're about to build, we can make a basic poll application which does:
- A public site that lets people view polls and vote in them.
- An admin site that lets us add, change and delete polls.
In this tutorial, we'll create our poll app right next to our manage.py file so that it can be imported as its own top-level module, rather than a submodule of mysite:
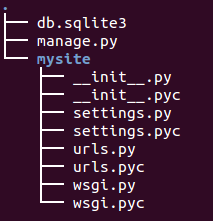
To create our app, type the following command:
$ python manage.py startapp polls
The command will create a directory polls, which is laid out like this:
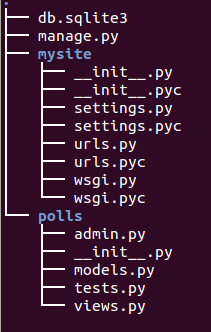
The first step in writing a database Web application in Django is to define our models - essentially, our database layout, with additional metadata.
In our simple poll application, we'll create two models:
- Poll has a question and a publication date.
- Choice has two fields: the text of the choice and a vote tally. Each Choice is associated with a Poll.
Edit the polls/models.py file so that it should look like this:
from django.db import models # Create your models here. class Poll(models.Model): question = models.CharField(max_length=200) pub_date = models.DateTimeField('date published') class Choice(models.Model): poll = models.ForeignKey(Poll) choice_text = models.CharField(max_length=200) votes = models.IntegerField(default=0)
Each model is represented by a class that subclasses django.db.models.Model. Each model has a number of class variables, each of which represents a database field in the model.
Each field is represented by an instance of a Field class - e.g., CharField for character fields and DateTimeField for datetimes. This tells Django what type of data each field holds.
The name of each Field instance (e.g. question or pub_date) is the field's name, in machine-friendly format. We'll use this value in our Python code, and our database will use it as the column name.
We can use an optional first positional argument to a Field to designate a human-readable name. That's used in a couple of introspective parts of Django, and it doubles as documentation. If this field isn't provided, Django will use the machine-readable name. In this example, we've only defined a human-readable name for Poll.pub_date. For all other fields in this model, the field's machine-readable name will suffice as its human-readable name.
Some Field classes have required arguments. CharField, for example, requires that we give it a max_length.
A Field can also have various optional arguments; in this case, we've set the default value of votes to 0.
Finally, note a relationship is defined, using ForeignKey. That tells Django each Choice is related to a single Poll. Django supports all the common database relationships: many-to-ones, many-to-manys and one-to-ones.
A model code enables Django to do:
- Create a database schema (CREATE TABLE statements).
- Create a Python database-access API for accessing Poll and Choice objects.
To be able to do that, we need to tell our project that the polls app is installed by editing the mysite/settings.py file to change the INSTALLED_APPS setting to include the string polls:
INSTALLED_APPS = ( 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'polls', )
Now Django knows to include the polls app.
Let's run python manage.py sql polls command:
$ ls db.sqlite3 manage.py mysite polls $ python manage.py sql polls BEGIN; CREATE TABLE "polls_poll" ( "id" integer NOT NULL PRIMARY KEY, "question" varchar(200) NOT NULL, "pub_date" datetime NOT NULL ) ; CREATE TABLE "polls_choice" ( "id" integer NOT NULL PRIMARY KEY, "poll_id" integer NOT NULL REFERENCES "polls_poll" ("id"), "choice_text" varchar(200) NOT NULL, "votes" integer NOT NULL ) ; COMMIT;
The table names are automatically generated by combining the name of the app (polls) and the lowercase name of the model - poll and choice.
- Primary keys (IDs) are added automatically. By convention, Django appends "_id" to the foreign key field name.
- The foreign key relationship is made explicit by a REFERENCES statement.
- It's tailored to the database we're using, so database-specific field types such as auto_increment (MySQL), serial (PostgreSQL), or integer primary key (SQLite) are handled for us automatically.
- The sql command doesn't actually run the SQL in our database - it just prints it to the screen so that we can see what SQL Django thinks is required. If we wanted to, we could copy and paste this SQL into our database prompt. However, as we will see shortly, Django provides an easier way of committing the SQL to the database.
We can also run the following commands:
- python manage.py validate - Checks for any errors in the construction of our models.
$ python manage.py validate 0 errors found
- python manage.py sqlcustom polls - Outputs any custom SQL statements (such as table modifications or constraints) that are defined for the application.
- python manage.py sqlclear polls - Outputs the necessary DROP TABLE statements for this app, according to which tables already exist in our database (if any).
- python manage.py sqlindexes polls - Outputs the CREATE INDEX statements for this app.
$ python manage.py sqlindexes polls BEGIN; CREATE INDEX "polls_choice_70f78e6b" ON "polls_choice" ("poll_id"); COMMIT;
- python manage.py sqlall polls - A combination of all the SQL from the sql, sqlcustom, and sqlindexes commands.
$ python manage.py sqlall polls BEGIN; CREATE TABLE "polls_poll" ( "id" integer NOT NULL PRIMARY KEY, "question" varchar(200) NOT NULL, "pub_date" datetime NOT NULL ) ; CREATE TABLE "polls_choice" ( "id" integer NOT NULL PRIMARY KEY, "poll_id" integer NOT NULL REFERENCES "polls_poll" ("id"), "choice_text" varchar(200) NOT NULL, "votes" integer NOT NULL ) ; CREATE INDEX "polls_choice_70f78e6b" ON "polls_choice" ("poll_id"); COMMIT;
Now, we need to run syncdb again to create those model tables in our database:
$ python manage.py syncdb Creating tables ... Creating table polls_poll Creating table polls_choice Installing custom SQL ... Installing indexes ... Installed 0 object(s) from 0 fixture(s)
The syncdb command runs the SQL from sqlall on our database for all apps in INSTALLED_APPS that don't already exist in our database. This creates all the tables, initial data and indexes for any apps we've added to our project since the last time we ran syncdb. The syncdb can be called as often as we like, and it will only ever create the tables that don't exist.
Now, play around with the free API Django gives us in the interactive Python shell. To invoke the Python shell, use this command:
$ python manage.py shell
We're using this instead of simply typing "python", because manage.py sets the DJANGO_SETTINGS_MODULE environment variable, which gives Django the Python import path to our mysite/settings.py file.
While we're in the shell, we can explore the database API:
>>> from polls.models import Poll, Choice # Import the model classes we just wrote. # No polls are in the system yet. >>> Poll.objects.all() [] # Create a new Poll. # Support for time zones is enabled in the default settings file, so # Django expects a datetime with tzinfo for pub_date. Use timezone.now() # instead of datetime.datetime.now() and it will do the right thing. >>> from django.utils import timezone >>> p = Poll(question="What's new?", pub_date=timezone.now()) # Save the object into the database. You have to call save() explicitly. >>> p.save() # Now it has an ID. Note that this might say "1L" instead of "1", depending # on which database you're using. That's no biggie; it just means your # database backend prefers to return integers as Python long integer # objects. >>> p.id 1 # Access database columns via Python attributes. >>> p.question "What's new?" >>> p.pub_date datetime.datetime(2014, 2, 11, 23, 19, 28, 437215, tzinfo=) # Change values by changing the attributes, then calling save(). >>> p.question = "What's up?" >>> p.save() # objects.all() displays all the polls in the database. >>> Poll.objects.all() [<Poll: Poll object>]
The <Poll: Poll object> is an unhelpful representation of this object. Let's fix that by editing the polls model (in the polls/models.py file) and adding a __unicode__() method to both Poll and Choice. (On Python 3, simply replace __unicode__() by __str__()).
from django.db import models # Create your models here. class Poll(models.Model): question = models.CharField(max_length=200) pub_date = models.DateTimeField('date published') def __unicode__(self): # Python 3: def __str__(self): return self.question class Choice(models.Model): poll = models.ForeignKey(Poll) choice_text = models.CharField(max_length=200) votes = models.IntegerField(default=0) def __unicode__(self): # Python 3: def __str__(self): return self.choice_text
We need to understand it's important to add __unicode__() to our models, not only for our own sanity when dealing with the interactive prompt, but also because objects' representations are used throughout Django's automatically-generated admin.
Just for demonstration, we may want to add a custom method:
from django.db import models import datetime from django.utils import timezone # Create your models here. class Poll(models.Model): question = models.CharField(max_length=200) pub_date = models.DateTimeField('date published') def __unicode__(self): # Python 3: def __str__(self): return self.question def was_published_recently(self): return self.pub_date >= timezone.now() - datetime.timedelta(days=1) class Choice(models.Model): poll = models.ForeignKey(Poll) choice_text = models.CharField(max_length=200) votes = models.IntegerField(default=0) def __unicode__(self): # Python 3: def __str__(self): return self.choice_text
We added import datetime and from django.utils import timezone, to reference Python's standard datetime module and Django's time-zone-related utilities in django.utils.timezone, respectively.
Now we may want to start a new Python interactive shell by running python manage.py shell again:
>>> from polls.models import Poll, Choice # Make sure our __unicode__() addition worked. >>> Poll.objects.all() [<Poll: What's up?>] # Django provides a rich database lookup API that's entirely driven by # keyword arguments. >>> Poll.objects.filter(id=1) [<Poll: What's up?>] >>> Poll.objects.filter(question__startswith='What') [<Poll: What's up?>] # Get the poll that was published this year. >>> from django.utils import timezone >>> current_year = timezone.now().year >>> Poll.objects.get(pub_date__year=current_year) <Poll: What's up?> # Request an ID that doesn't exist, this will raise an exception. >>> Poll.objects.get(id=2) Traceback (most recent call last): ... DoesNotExist: Poll matching query does not exist. Lookup parameters were {'id': 2} # Lookup by a primary key is the most common case, so Django provides a # shortcut for primary-key exact lookups. # The following is identical to Poll.objects.get(id=1). >>> Poll.objects.get(pk=1) <Poll: What's up?> # Make sure our custom method worked. >>> p = Poll.objects.get(pk=1) >>> p.was_published_recently() True # Give the Poll a couple of Choices. The create call constructs a new # Choice object, does the INSERT statement, adds the choice to the set # of available choices and returns the new Choice object. Django creates # a set to hold the "other side" of a ForeignKey relation # (e.g. a poll's choices) which can be accessed via the API. >>> p = Poll.objects.get(pk=1) # Display any choices from the related object set -- none so far. >>> p.choice_set.all() [] # Create three choices. >>> p.choice_set.create(choice_text='Not much', votes=0) <Choice: Not much> >>> p.choice_set.create(choice_text='The sky', votes=0) <Choice: The sky> >>> c = p.choice_set.create(choice_text='Just hacking again', votes=0) # Choice objects have API access to their related Poll objects. >>> c.poll <Poll: What's up?> # And vice versa: Poll objects get access to Choice objects. >>> p.choice_set.all() [<Choice: Not much>, <Choice: The sky>, <Choice: Just hacking again>] >>> p.choice_set.count() 3 # The API automatically follows relationships as far as you need. # Use double underscores to separate relationships. # This works as many levels deep as you want; there's no limit. # Find all Choices for any poll whose pub_date is in this year # (reusing the 'current_year' variable we created above). >>> Choice.objects.filter(poll__pub_date__year=current_year) [<Choice: Not much>, <Choice: The sky>, <Choice: Just hacking again>] # Let's delete one of the choices. Use delete() for that. >>> c = p.choice_set.filter(choice_text__startswith='Just hacking') >>> c.delete()
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization