Authentication (User Registration B - Customized Form) on Shared Host using FastCGI
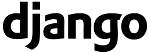
As a continuation from the previous chapter, User Registration on Shared Host using FastCGI. in this chapter, we will extend user registration. It will include email data and it's a customized version of UserCreationForm.
As in the previous chapters, we need keep two files in ~/www/dj directory:
# pwd /home2/bogotob1/public_html/dj # ls ./ ../ djangoproject.fcgi* .htaccess
djangoproject.fcgi:
#! /home2/bogotob1/python/bin/python import sys, os sys.path.insert(0, "/home2/bogotob1/python") sys.path.insert(13, "/home2/bogotob1/djangoproject") os.environ['DJANGO_SETTINGS_MODULE'] = 'djangoproject.settings' from django.core.servers.fastcgi import runfastcgi runfastcgi(method="threaded", daemonize="false")
where /home2/bogotob1/ is my home(~) directory.
.htaccess:
# django AddHandler fcgid-script .fcgi RewriteEngine On RewriteCond %{REQUEST_FILENAME} !-f RewriteRule ^(.*)$ djangoproject.fcgi/$1 [QSA,L]
Similar to the urls, we're going to add new views for registration to views.py. We'll also make a customized form (forms.py) by extending the UserCreationForm imported from django.contrib.auth.forms.
from django import forms from django.contrib.auth.models import User from django.contrib.auth.forms import UserCreationForm class MyRegistrationForm(UserCreationForm): email = forms.EmailField(required = True) class Meta: model = User fields = ('username', 'email', 'password1', 'password2') def save(self, commit = True): user = super(UserCreationForm, self).save(commit = False) user.email = self.cleaned_data['email'] if commit: user.save() return user
Now that we have a customized form, we're going to use that form for registration. Here is a new views.py:
from django.shortcuts import render_to_response from django.http import HttpResponseRedirect from django.contrib import auth from django.core.context_processors import csrf from forms import MyRegistrationForm def home(request): return render_to_response('home.html', {'Testing' : 'Django Template Inheritance ', 'HelloHello' : 'Hello World - Django'}) def login(request): c = {} c.update(csrf(request)) return render_to_response('login.html', c) def auth_view(request): username = request.POST.get('username', '') password = request.POST.get('password', '') user = auth.authenticate(username=username, password=password) if user is not None: auth.login(request, user) return HttpResponseRedirect('/dj/accounts/loggedin') else: return HttpResponseRedirect('/dj/accounts/invalid') def logout(request): auth.logout(request) return render_to_response('logout.html') def loggedin(request): return render_to_response('loggedin.html', {'full_name': request.user.username}) def invalid_login(request): return render_to_response('invalid_login.html') def register_user(request): # 2nd time around if request.method == 'POST': form = MyRegistrationForm(request.POST) if form.is_valid(): form.save() return HttpResponseRedirect('/dj/accounts/register_success') # 1st time visit args = {} args.update(csrf(request)) # form with no input args['form'] = MyRegistrationForm() print args return render_to_response('register.html', args) def register_success(request): return render_to_response('register_success.html')
The same urls.py we used before:
from django.conf.urls import patterns, include, url from django.contrib import admin admin.autodiscover() urlpatterns = patterns('', # Examples: url(r'^$', 'djangoapp.views.home', name='home'), # url(r'^blog/', include('blog.urls')), url(r'^admin/', include(admin.site.urls)), # user authentication urls url(r'^accounts/login/$', 'djangoapp.views.login'), url(r'^accounts/auth/$', 'djangoapp.views.auth_view'), url(r'^accounts/login/accounts/auth/$', 'djangoapp.views.auth_view'), url(r'^accounts/logout/$', 'djangoapp.views.logout'), url(r'^accounts/loggedin/$', 'djangoapp.views.loggedin'), url(r'^accounts/invalid/$', 'djangoapp.views.invalid_login'), url(r'^accounts/register/$', 'djangoapp.views.register_user'), url(r'^accounts/register_success/$', 'djangoapp.views.register_success'), )
Here is the tree view of the files:
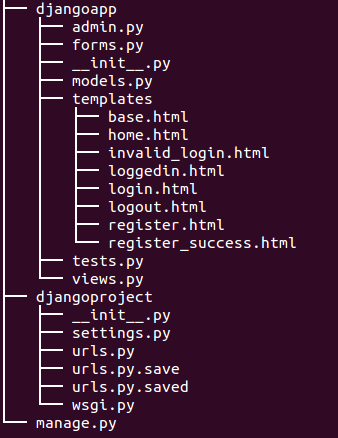
Let's register with username as register_test2:
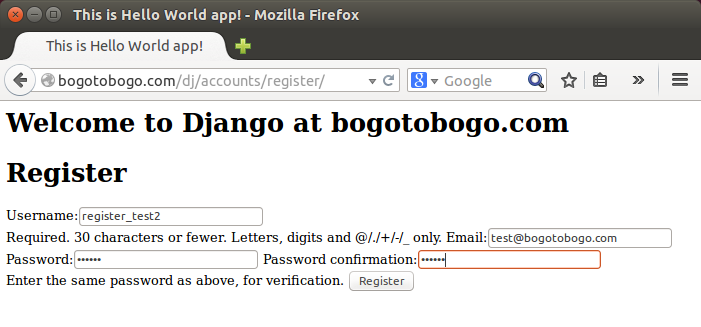
If the registration is successful, we get the following page:
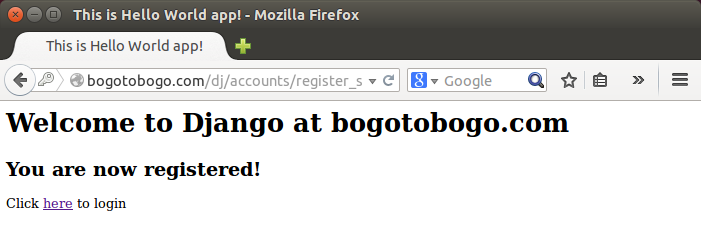
We can check if the user (register_test) is there from admin page:
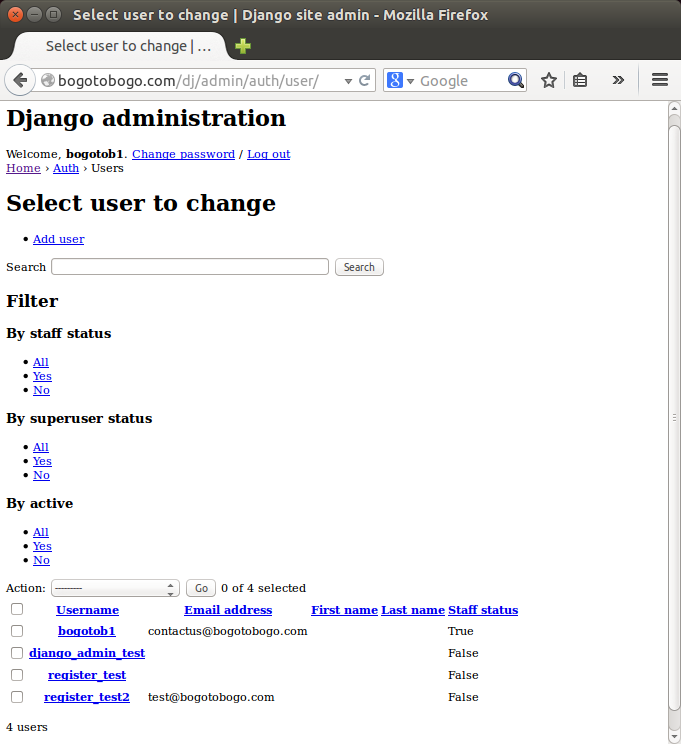
To add first and last names for user registration is straight forward. We just modify the forms.py:
from django import forms from django.contrib.auth.models import User from django.contrib.auth.forms import UserCreationForm class MyRegistrationForm(UserCreationForm): email = forms.EmailField(required = True) first_name = forms.CharField(required = True) last_name = forms.CharField(required = True) class Meta: model = User fields = ('last_name', 'first_name', 'username', 'email', 'password1', 'password2') def save(self, commit = True): user = super(UserCreationForm, self).save(commit = False) user.email = self.cleaned_data['email'] user.first_name = self.cleaned_data['first_name'] user.last_name = self.cleaned_data['last_name'] if commit: user.save() return user
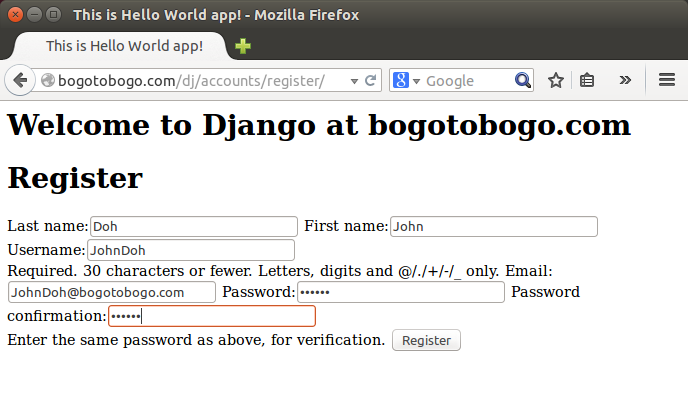
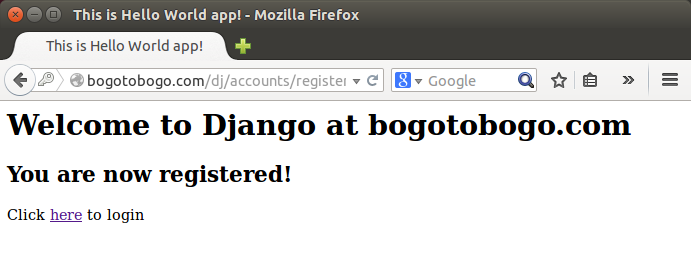
We can check MySQL database if the user is registered via phpMyAdmin in addition to the DjangoAdmin:
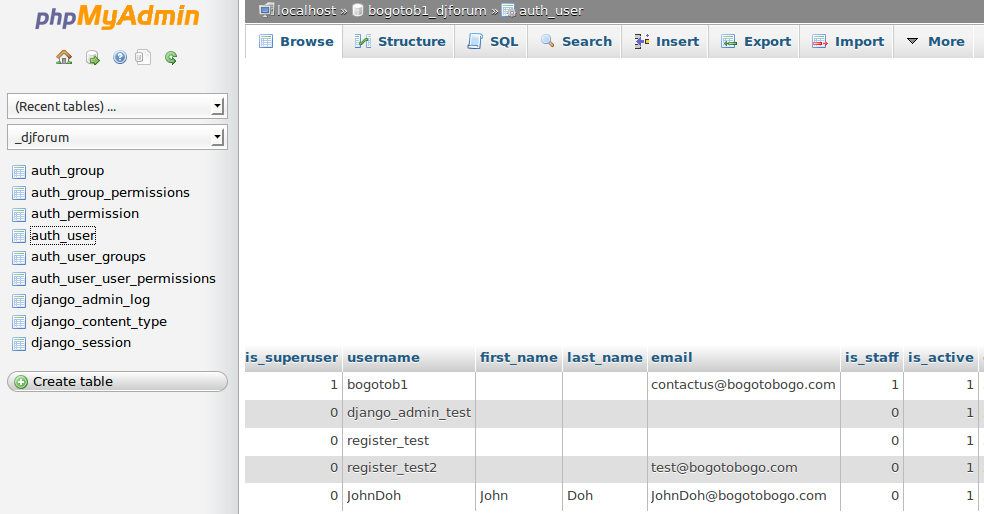
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization