16. Blog app : views and urls with template
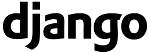
Here is the current project file structure:
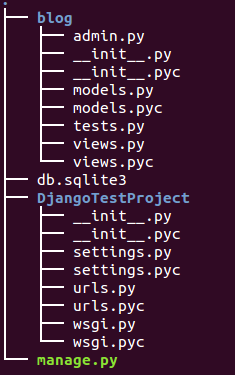
First, we creates a templates directory.
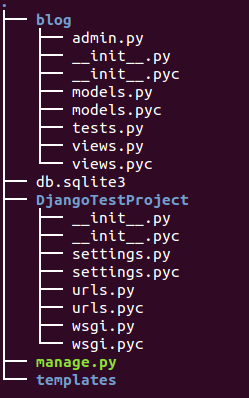
We also need template file, hello.html under tempaltes directory:
<html> <body> Hi {{name}}, from templates/hello.html </body> </html>
Now, how do we tell Django where the templates are?
settings.py!
Add the following lines to settings.py:
TEMPLATE_DIRS = ( '/home/k/Django/DjangoVirtualenv/DjangoTestProject/templates', )
We specified the absolute path to the templates. That's the way of telling Django the location of the templates.
from django.http import HttpResponse from django.template.loader import get_template from django.template import Context # Create your views here. def hello(request): name = "Bogo" html = "<html><body>Hi %s from views.py. </body></html>" %name return HttpResponse(html) def hello_template(request): name = "Bogo" tp = get_template("hello.html") html = tp.render(Context({'name': name})) return HttpResponse(html)
The django.template.loader has two functions to load templates from files. One of them is get_template(), and the syntax looks like this:
get_template(template_name[, dirs])
get_template() returns the compiled template (a Template object) for the template with the given name. If the template doesn't exist, it raises django.template.TemplateDoesNotExist.
Once we have a compiled Template object, we can render a context with it. The Context class lives at django.template.Context. We usually instantiate Context objects by passing in a fully-populated dictionary to Context().
Now, we have two ways of display:
- blog.views.hello: The simplest - html string without template
- blog.views.hello_template: Not the simplest - using template
from django.conf.urls import patterns, include, url #from django.contrib import admin #admin.autodiscover() urlpatterns = patterns('', # Examples: # url(r'^$', 'DjangoTestProject.views.home', name='home'), # url(r'^blog/', include('blog.urls')), #url(r'^admin/', include(admin.site.urls)), url(r'^hello/', 'blog.views.hello'), url(r'^hello_template/', 'blog.views.hello_template'), )
$ python manage.py runserver
We can see both of the displays are working!
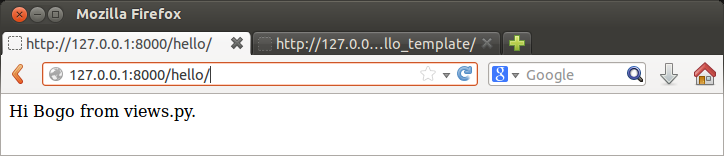
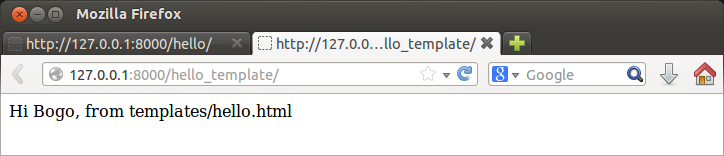
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization