8. Django 1.8 Server Build - CentOS 7 hosted on VPS - Model 3 (using shell)
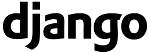
In previous chapter (7. Django 1.8 Server Build - CentOS 7 hosted on VPS - Model 2 : populate tables, list_display, and search_fields), we populated tables, and then added more display. Also, search feature has been added.
In this chapter, we'll use shell to update our models and views.
Let's start using shell:
$ ls car djangotest manage.py [sfvue@sf djangotest]$ python manage.py shell Python 2.7.5 (default, Jun 17 2014, 18:11:42) [GCC 4.8.2 20140120 (Red Hat 4.8.2-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. (InteractiveConsole) >>>
If we want to access models, we need to import them:
>>> from car.models import Car, Make
We can check our classes:
>>> from car.models import Car, Make >>> Car <class 'car.models.Car'> >>> Make <class 'car.models.Make'> >>>
To see what we can do with Car, we can use dir():
>>> dir(Car) ['DoesNotExist', 'MultipleObjectsReturned', '__class__', '__delattr__', '__dict__', '__doc__', '__eq__', '__format__', '__getattribute__', '__hash__', '__init__', u'__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setstate__', '__sizeof__', '__str__', '__subclasshook__', '__unicode__', '__weakref__', '_base_manager', '_check_column_name_clashes', '_check_field_name_clashes', '_check_fields', '_check_id_field', '_check_index_together', '_check_local_fields', '_check_long_column_names', '_check_m2m_through_same_relationship', '_check_managers', '_check_model', '_check_ordering', '_check_swappable', '_check_unique_together', '_default_manager', '_deferred', '_do_insert', '_do_update', '_get_FIELD_display', '_get_next_or_previous_by_FIELD', '_get_next_or_previous_in_order', '_get_pk_val', '_get_unique_checks', '_meta', '_perform_date_checks', '_perform_unique_checks', '_save_parents', '_save_table', '_set_pk_val', 'check', 'clean', 'clean_fields', 'date_error_message', 'delete', 'from_db', 'full_clean', 'get_deferred_fields', 'get_locality_display', 'make', 'objects', 'pk', 'prepare_database_save', 'refresh_from_db', 'save', 'save_base', 'serializable_value', 'unique_error_message', 'validate_unique'] >>>
But we usually use objects when we dealing with classes, so:
>>> dir(Car.objects) ['__class__', '__delattr__', '__dict__', '__doc__', '__eq__', '__format__', '__getattribute__', '__hash__', '__init__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__unicode__', '__weakref__', '_constructor_args', '_copy_to_model', '_db', '_get_queryset_methods', '_hints', '_inherited', '_insert', '_queryset_class', '_set_creation_counter', '_update', 'aggregate', 'all', 'annotate', 'bulk_create', 'check', 'complex_filter', 'contribute_to_class', 'count', 'create', 'creation_counter', 'dates', 'datetimes', 'db', 'db_manager', 'deconstruct', 'defer', 'distinct', 'earliest', 'exclude', 'exists', 'extra', 'filter', 'first', 'from_queryset', 'get', 'get_or_create', 'get_queryset', 'in_bulk', 'iterator', 'last', 'latest', 'model', 'name', 'none', 'only', 'order_by', 'prefetch_related', 'raw', 'reverse', 'select_for_update', 'select_related', 'update', 'update_or_create', 'use_in_migrations', 'using', 'values', 'values_list'] >>>
To see what object we have now:
>>> Car.objects.all() [<Car: M6 Coupe>, <Car: M6 Convertible 2013>, <Car: M6 Coupe 2014>, <Car: Tesla Roadster 2011>]
Note that we can make the output as a zero based array:
>>> cars = Car.objects.all() >>> cars [<Car: M6 Coupe>, <Car: M6 Convertible 2013>, <Car: M6 Coupe 2014>, <Car: Tesla Roadster 2011>]a
Now, we can access individual object. For example, "M6 Coupe":
>>> m6 = cars[0] >>> m6 <Car: M6 Coupe>
Let's check what we can do with "m6":
>>> dir(m6) ['DoesNotExist', 'MultipleObjectsReturned', '__class__', '__delattr__', '__dict__', '__doc__', '__eq__', '__format__', '__getattribute__', '__hash__', '__init__', u'__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setstate__', '__sizeof__', '__str__', '__subclasshook__', '__unicode__', '__weakref__', '_base_manager', '_check_column_name_clashes', '_check_field_name_clashes', '_check_fields', '_check_id_field', '_check_index_together', '_check_local_fields', '_check_long_column_names', '_check_m2m_through_same_relationship', '_check_managers', '_check_model', '_check_ordering', '_check_swappable', '_check_unique_together', '_default_manager', '_deferred', '_do_insert', '_do_update', '_get_FIELD_display', '_get_next_or_previous_by_FIELD', '_get_next_or_previous_in_order', '_get_pk_val', '_get_unique_checks', '_make_cache', '_meta', '_perform_date_checks', '_perform_unique_checks', '_save_parents', '_save_table', '_set_pk_val', '_state', 'check', 'clean', 'clean_fields', 'date_error_message', 'delete', 'description', 'from_db', 'full_clean', 'get_deferred_fields', 'get_locality_display', 'id', 'locality', 'make', 'make_id', 'name', 'objects', 'pk', 'prepare_database_save', 'refresh_from_db', 'save', 'save_base', 'serializable_value', 'slug', 'unique_error_message', 'validate_unique'] >>> m6.slug u'BMW-M6-F12-Coupe-2015' >>> m6.make <Make: BMW>
Let's list all cars and makes:
>>> for c in cars: ... c ... <Car: M6 Coupe> <Car: M6 Convertible 2013> <Car: M6 Coupe 2014> <Car: Tesla Roadster 2011> >>> makes = Make.objects.all() >>> for m in makes: ... m ... <Make: BMW> <Make: Tesla>
Now, let's try to use filters whether a car is "Domestic" or "Import":
>>> Car.objects.filter(locality='I') [<Car: M6 Coupe>, <Car: M6 Convertible 2013>, <Car: M6 Coupe 2014>] >>> Car.objects.filter(locality='D') [<Car: Tesla Roadster 2011>] >>>
We can also use get() to retrieve single object:
>>> Car.objects.get(slug='BMW-M6-F12-Coupe-2015') <Car: M6 Coupe>
However, when we use the get() method, we should be sure the uniqueness of the filter, otherwise we get an error "MultipleObjectsReturned" as in the following example:
>>> Car.objects.get(locality='I') Traceback (most recent call last): File "<console>", line 1, inFile "/usr/lib64/python2.7/site-packages/django/db/models/manager.py", line 127, in manager_method return getattr(self.get_queryset(), name)(*args, **kwargs) File "/usr/lib64/python2.7/site-packages/django/db/models/query.py", line 338, in get (self.model._meta.object_name, num) MultipleObjectsReturned: get() returned more than one Car -- it returned 3! >>>
Note also that every object gets primary key automatically:
>>> Car.objects.get(slug='BMW-M6-F12-Coupe-2015') <Car: M6 Coupe> >>> Car.objects.get(slug='BMW-M6-F12-Coupe-2015').pk 1L >>> Car.objects.get(pk=1) <Car: M6 Coupe> >>>
There are all different kinds of ways that we can interface with all the objects that are stored in our models. The materials we learned in the tutorial will be the basis of linking models to views. We'll do almost the same operations when we deal with views.
Continue : 9. Views (templates and css)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization